Beginner and advanced examples of Stacking in Numpy - Learn how to join a sequence of arrays easily.
Numpy is an amazing library for data science and machine learning, so there’s no way around it if you want to become a data professional. Mastering the ins and outs of the package is mandatory because there’s no point in reinventing the wheel - pretty much anything you can think of has already been implemented.
Today you’ll learn all about np stack - or the Numpy’s stack()
function. Put simply, it allows you to join arrays row-wise (default) or column-wise, depending on the parameter values you specify. We’ll go over the fundamentals and the function signature, and then jump into examples in Python.
What is np stack?
Numpy’s np stack function is used to stack/join arrays along a new axis. It will return a single array as a result of stacking multiple sequences with the same shape. You can stack multidimensional arrays as well, and you’ll learn how shortly.
But first, let’s explain the difference between horizontal and vertical stacking.
Horizontal vs. vertical stack in Numpy
Stacking arrays horizontally means that you take arrays of the same dimensions and stack them on top of each other. Each input array will be a row in the resulting array.
Take a look at the following image for a better understanding:
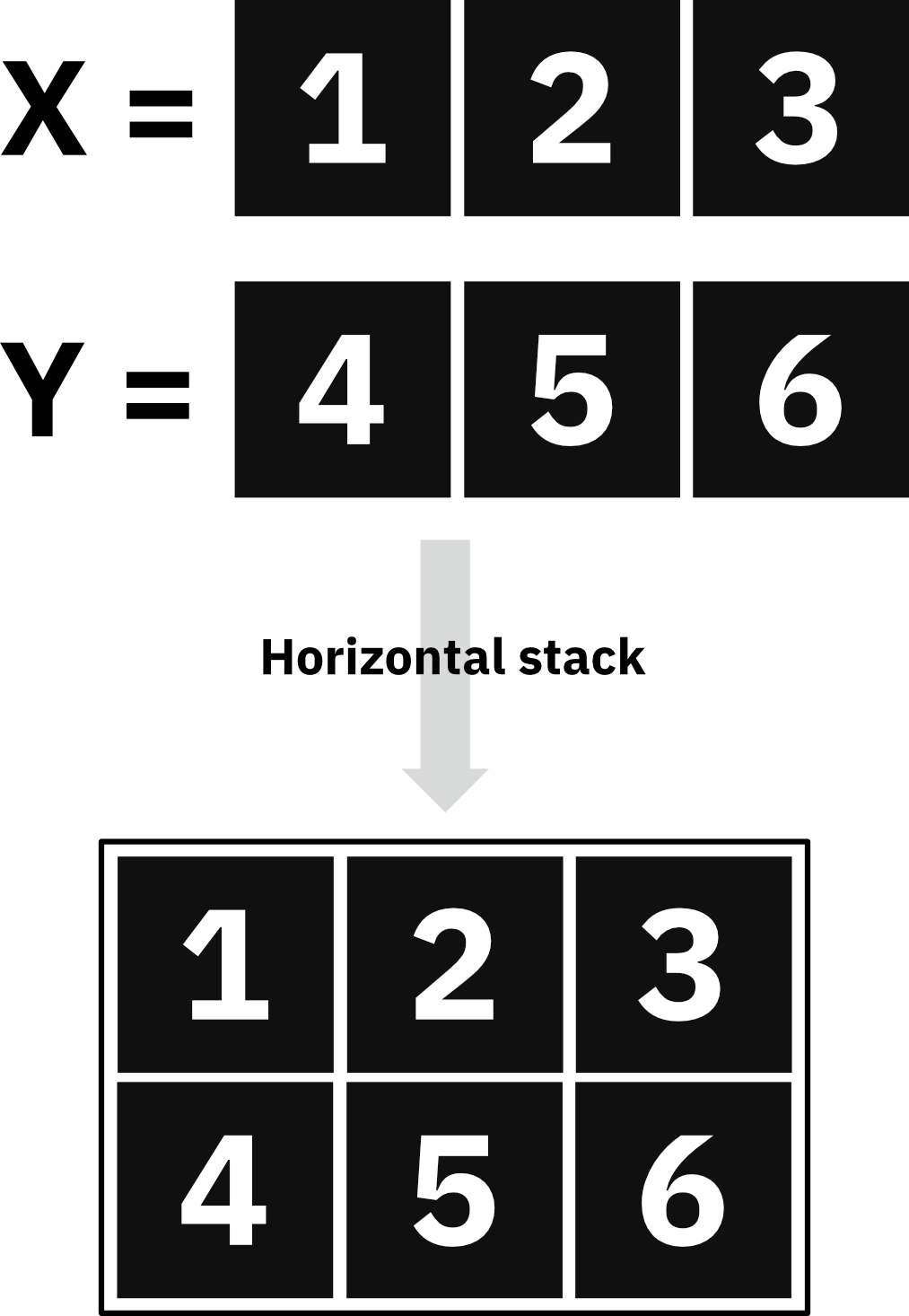
Image 1 - Horizontal stacking explained (image by author)
Vertical stacking works just the opposite. One row of two vertically stacked arrays contains corresponding elements from both.
For example, the first row of a vertically stacked array Z will contain the first elements of the input arrays X and Y.
Maybe you’ll find it easier to grasp visually:
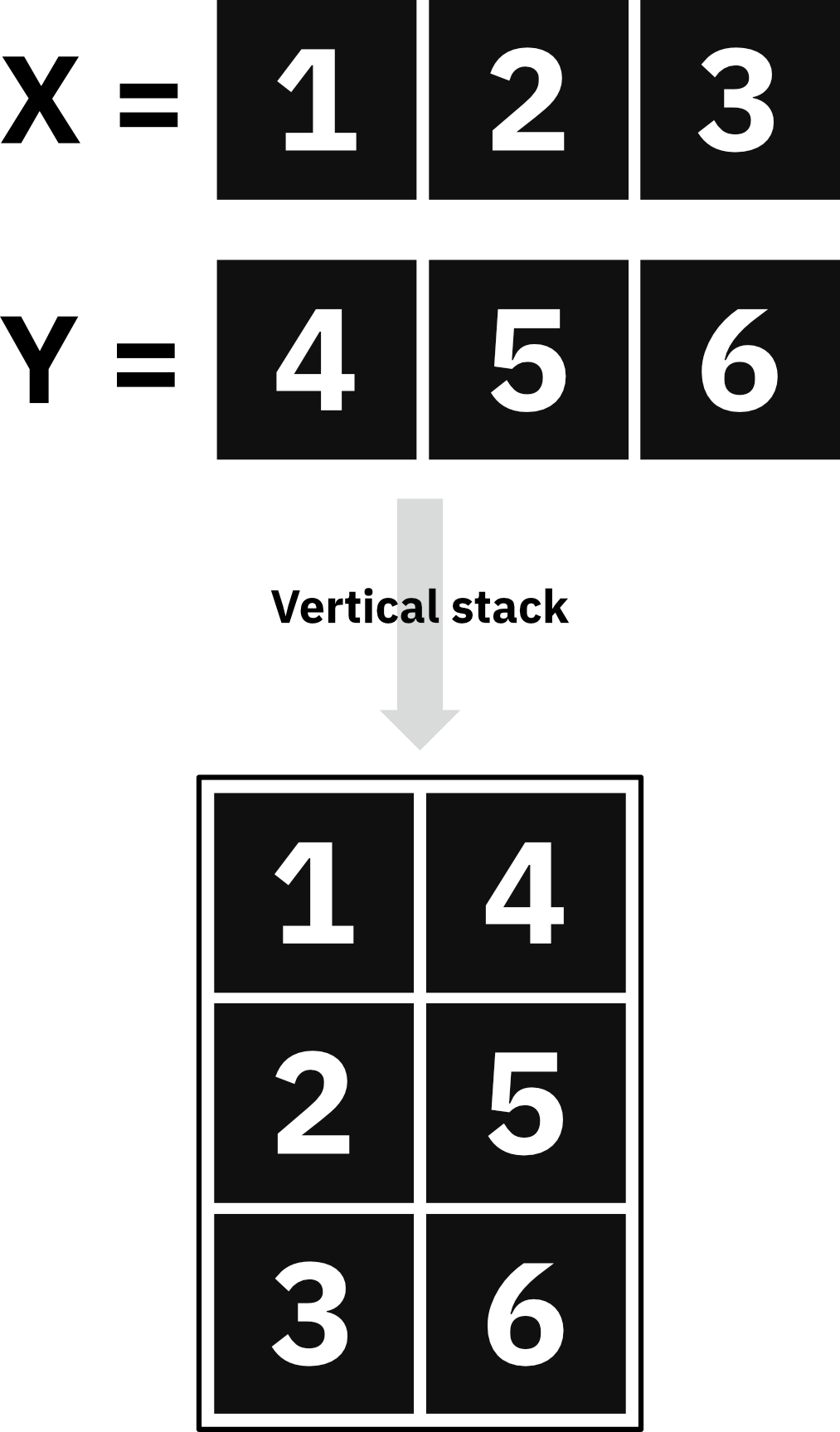
Image 2 - Vertical stacking explained (image by author)
And with that out the way, let’s go over the np stack function signature.
Function parameters explained
The np stack function can take up to three parameters, of which only the first one is mandatory:
arrays
- sequence of arrays, or array of arrays you want to stackaxis
- integer, the axis along which you want to stack the arrays (0 = row-wise stacking, 1 = column-wise stacking for 1D arrays, or use -1 to use the last axis)out
- optional destination to place the results. If provided, the output array shape must match the stacking result shape
Enough theory! Let’s now go over some practical examples.
Numpy Stack in Action - Function Examples
We’ve talked a lot about horizontal and vertical stacking, so let’s see how it works in practice.
Numpy horizontal stacking (row-wise)
To stack two numpy arrays horizontally, you just need to call the np.stack function and pass in the arrays. No other parameters are required:
import numpy as np
arr1 = np.array([1, 2, 3, 4])
arr2 = np.array([5, 6, 7, 8])
# Horizontal (row-wise) stacking #1
arr_stacked = np.stack([arr1, arr2])
print('Numpy horizontal stacking method #1')
print('-----------------------------------')
print(arr_stacked)
Here’s the resulting output:
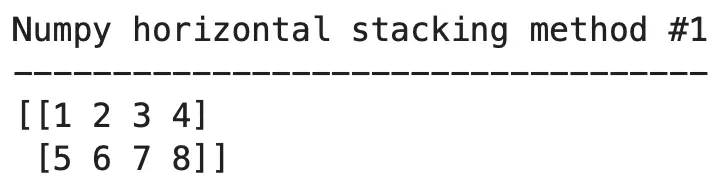
Image 3 - Horizontal stacking in Numpy (1) (image by author)
As you can see, the output looks a lot like a Numpy version of a Pandas DataFrame, which means one array pretty much equals one row of the matrix.
To be more explicit, you can achieve the same results by writing axis=0
as the second parameter:
import numpy as np
arr1 = np.array([1, 2, 3, 4])
arr2 = np.array([5, 6, 7, 8])
# Horizontal (row-wise) stacking #2
arr_stacked = np.stack([arr1, arr2], axis=0)
print('Numpy horizontal stacking method #2')
print('-----------------------------------')
print(arr_stacked)
The results are identical:
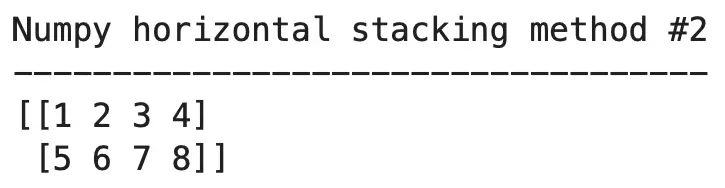
Image 4 - Horizontal stacking in Numpy (2) (image by author)
Next, let’s explore vertical stacking.
Numpy vertical stacking (column-wise)
To stack two numpy arrays vertically, just change the value of the axis
parameter to 1:
import numpy as np
arr1 = np.array([1, 2, 3, 4])
arr2 = np.array([5, 6, 7, 8])
# Vertical (column-wise) stacking #1
arr_stacked = np.stack([arr1, arr2], axis=1)
print('Numpy vertical stacking method #1')
print('---------------------------------')
print(arr_stacked)
Now the arrays are stacked as columns, meaning you’ll have as many columns as you have provided arrays:
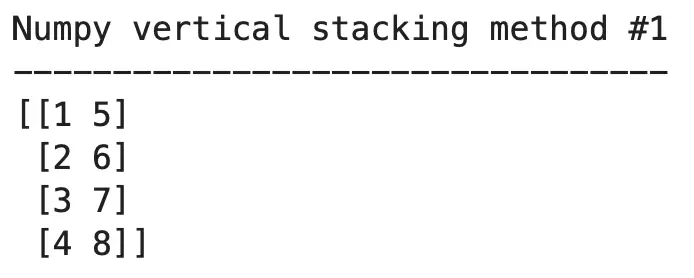
Image 5 - Vertical stacking in Numpy (1) (image by author)
With simple 1D arrays, you can also set axis=-1
to stack the arrays vertically:
import numpy as np
arr1 = np.array([1, 2, 3, 4])
arr2 = np.array([5, 6, 7, 8])
# Vertical (column-wise) stacking #2
arr_stacked = np.stack([arr1, arr2], axis=-1)
print('Numpy vertical stacking method #2')
print('---------------------------------')
print(arr_stacked)
The results are identical:
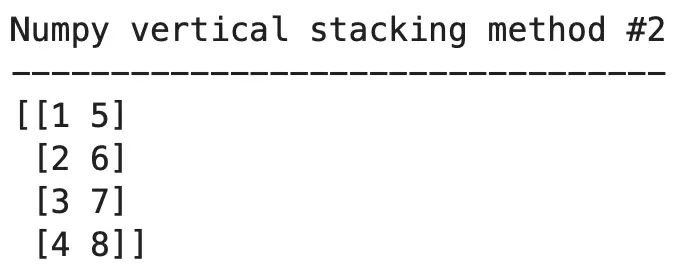
Image 6 - Vertical stacking in Numpy (2) (image by author)
Next, let’s discuss some more on stacking N-dimensional arrays.
Join 1D arrays with stack()
You’ve already seen how to stack 1-dimensional arrays, but here’s a recap:
import numpy as np
arr1 = np.array([1, 2, 3, 4])
arr2 = np.array([5, 6, 7, 8])
# Stacking 1D arrays
arr_stacked = np.stack([arr1, arr2])
print('Numpy stacking 1D arrays')
print('------------------------')
print(arr_stacked)
And the output:
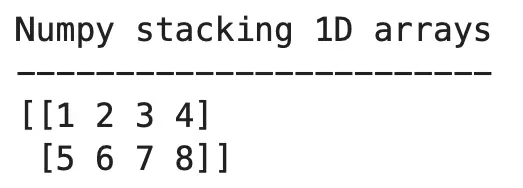
Image 7 - Stacking 1D arrays (image by author)
Remember that you can change the value of the axis
parameter if you want to stack the arrays column-wise.
Join 2D arrays with stack()
The procedure is identical for stacking 2D arrays with np stack. Here’s an example:
import numpy as np
arr1 = np.array([
[1, 2, 3, 4],
[5, 6, 7, 8]
])
arr2 = np.array([
[9, 10, 11, 12],
[13, 14, 15, 16]
])
# Stacking 2D arrays #1
arr_stacked = np.stack([arr1, arr2])
print('Numpy stacking 2D arrays method #1')
print('----------------------------------')
print(arr_stacked)
We now get a 3-dimensional array back, each element being a 2-dimensional array of two horizontally stacked arrays:
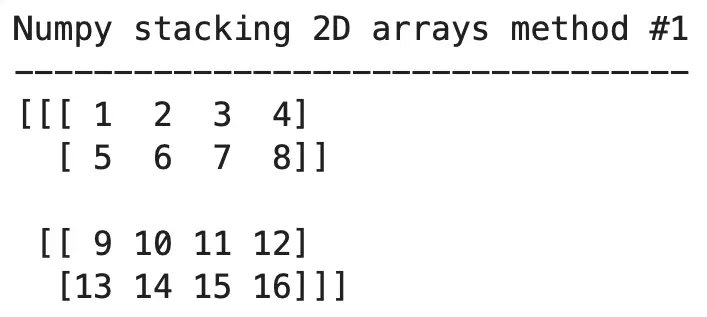
Image 8 - Stacking 2D arrays (1) (image by author)
As per usual, you can stack 2-dimensional arrays vertically:
import numpy as np
arr1 = np.array([
[1, 2, 3, 4],
[5, 6, 7, 8]
])
arr2 = np.array([
[9, 10, 11, 12],
[13, 14, 15, 16]
])
# Stacking 2D arrays #2
arr_stacked = np.stack([arr1, arr2], axis=1)
print('Numpy stacking 2D arrays method #2')
print('----------------------------------')
print(arr_stacked)
Here’s the output:
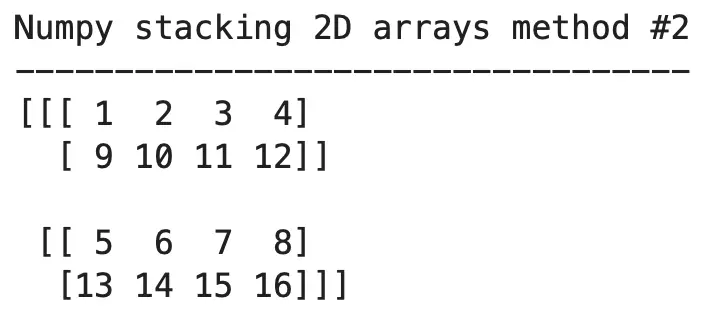
Image 9 - Stacking 2D arrays (2) (image by author)
That’s pretty much all there is to numpy stacking, at least for the basics. Up next, we’ll go over some advanced usage examples and frequently asked questions.
Advanced: np stack in a loop
One of the commonly asked questions is how can you use np stack in a loop. Here’s an example - it will first combine two 2-dimensional arrays into a 3-dimensional one:
import numpy as np
arr1 = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9], [10, 11, 12]])
arr2 = np.array([[13, 14, 15], [16, 17, 18]])
matrix = [arr1, arr2]
print('Numpy stacking in a loop - intermediary matrix')
print('----------------------------------------------')
print(matrix)
This is the intermediary output:
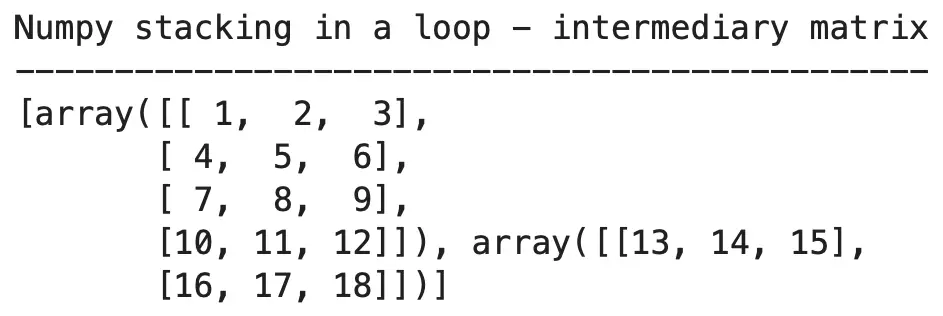
Image 10 - Numpy stacking in a loop (1) (image by author)
And now to produce a single 2-dimensional array with the elements stacked horizontally, you can use a loop:
arr3 = np.empty(shape=[0, matrix[0].shape[1]])
for m in matrix:
arr3 = np.append(arr3, m, axis=0)
print('Numpy stacking in a loop')
print('------------------------')
print(arr3)
Here’s the result:
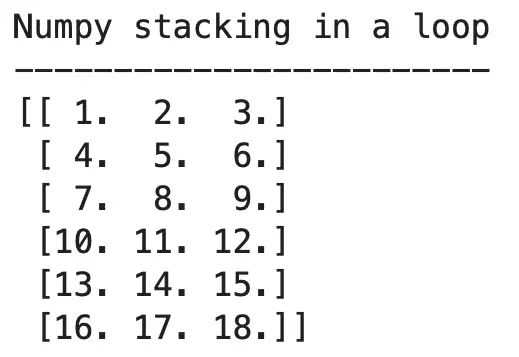
Image 11 - Numpy stacking in a loop (2) (image by author)
We’ll now cover some frequently asked questions about the np stack function in Python.
FAQ
What is the difference between stack and concatenate?
Put simply, np stack function will return a 2D array when two 1D arrays are passed in. The np concatenate function takes elements of all input arrays and returns them as a single 1D array.
What is numpy dstack?
The numpy dstack function allows you to combine arrays index by index and store the results like a stack. Here’s an example:
import numpy as np
arr1 = np.array([1, 2, 3, 4])
arr2 = np.array([5, 6, 7, 8])
# Numpy depth stacking - dstack
arr_stacked = np.dstack([arr1, arr2])
print('Numpy depth stacking')
print('--------------------')
print(arr_stacked)
print()
print(f'Shape = {arr_stacked.shape}')
And the output:
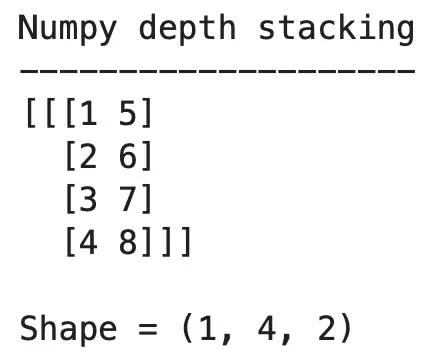
Image 12 - Numpy dstack (image by author)
So, we had two 1x4 arrays coming in, and dstack
combined them vertically into a 3-dimensional array format. Neat for some use cases.