Python For Loop Range
Everyone and their grandmothers know about Python’s for
loop. But did you know you can combine it with the built-in range()
function to simplify iterating over a range of numbers? If you didn’t, you’re in luck, because today you’ll learn the ins and outs of Python for loop range syntax.
Working with nested Python dictionaries used to be tricky and time-consuming. Well, not anymore!
We’ll start simple with the basic range iteration examples, and then dive deeper into more advanced use cases and real-world applications, such as iterating through a Python list with a Python for loop range, reversing the range order, reversing a list, and much more.
Let’s get started!
Table of contents:
Python For Loop Range - How to Use the range() Function
The range()
function in Python allows you to generate a sequence of numbers. It’s commonly used in for
loops to iterate over a specific range of values. The range()
function accepts one, two, or three arguments: start
, stop
, and step
. These aren’t named arguments, so specify only the values when working with range()
.
Let’s go over each of them next.
range(stop)
When you use range(stop)
, the function will generate a sequence of numbers starting from 0 and ending at stop - 1
. It increments the value by 1 at each iteration. Here’s an example:
for i in range(5):
print(i, end=" ")
Here’s the output you should see:
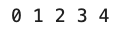
Image 1 - Python for Loop Range with stop argument (image by author)
In a nutshell, use this syntax if you only care about the stop value, and are fine with starting at zero.
range(start, stop)
By using range(start, stop)
, you can define starting and stopping point for the sequence. The range()
function generates numbers starting from start
and stops before reaching stop
. Let’s see an example:
for i in range(2, 7):
print(i, end=" ")
And here’s the output:
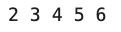
Image 2 - Python for Loop Range with stop and stop arguments (image by author)
Values from 2 to 6 are printed, since range()
stops before the stop
argument. Keep that in mind.
range(start, stop, step)
Adding a third argument, step
, allows you to specify the increment between each number in the sequence. The step
value can be positive or negative. We’ll cover only the positive value in this section and will focus on the negative extensively later.
Consider the following example:
for i in range(1, 10, 2):
print(i, end=" ")
Here’s the output:
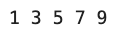
Image 3 - Python for Loop Range with start, stop, and step arguments (image by author)
As you can see, the range()
function now generates numbers from 1 to 10 (not included) with a step of 2. Neat use case if you need only the odd numbers in some range.
How to Iterate Through a Python List with Python For Loop Range
The for
loop, when combined with the range()
function, provides a convenient way to iterate through a Python list. You can utilize the index values provided by the range()
function to access and manipulate individual elements of the list.
Consider the following example in which we have a list of employees and want to print each of them one by one:
employees = ["Bob", "Mark", "Jane", "Ingrid"]
for i in range(len(employees)):
print(employees[i])
This is the output you will see:
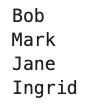
Image 4 - Iterating through a list with range() (image by author)
In the above example, we use the len()
function to determine the length of the employee list. The range()
function generates a sequence of index values corresponding to the length of the list. By iterating over these indices, we can access each element of the list using indexing (employees[i]
) within the for
loop.
As with almost anything in Python, there’s a simpler approach.
The alternative is to directly iterate over the list itself:
employees = ["Bob", "Mark", "Jane", "Ingrid"]
for employee in employees:
print(employee)
The output is identical to the one we had before:
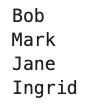
Image 5 - Iterating through a list with range() (2) (image by author)
In this case, the for
loop directly iterates over the elements of the employee list, eliminating the need for indexing. This approach is more readable and convenient because you don’t need to manipulate the index values.
Up next, let’s go over reversing the range in Python.
How to Reverse the Range Order in Python
There are situations where you need to iterate through a range in reverse order. Python provides a couple of approaches to achieve this: by using the reversed()
function or utilizing a negative step value within the range()
function.
Let’s go over each next.
Using the reversed() Function
The reversed()
function allows you to reverse any iterable object, including a range. By wrapping the range()
function with reversed()
, you can effortlessly iterate through the range in reverse order.
Consider the following example:
for i in reversed(range(5)):
print(i, end=" ")
Our numbers are now printed from 4 to 0:
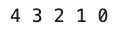
Image 6 - Python reversed() function (image by author)
In the above example, range(5)
generates a sequence of numbers from 0 to 4. By passing this range to the reversed()
function, the order of iteration is reversed, resulting in a reverse range from 4 to 0.
Using Negative Step
The alternative approach is to use the negative step value within the range()
function. This will essentially count down from the starting to the stopping point. The syntax might look strange at first since you have to specify at least one negative value (step
).
Here’s an example:
for i in range(4, -1, -1):
print(i, end=" ")
This is the output you should see:
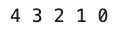
Image 7 - Python range() negative step function (image by author)
In this example, range(4, -1, -1)
generated a range that starts from 4, decrements by 1 at each iteration, and stops before reaching -1.
Up next, let’s apply this knowledge to a practical Python problem - list reversal.
How to Reverse a List with Python For Loop Range
In addition to iterating through a range in reverse order, you may also need to reverse the order of the elements within a list itself. Python’s for
loop in conjunction with the range()
function can be utilized to accomplish this task efficiently.
Take a look at the following example - it declares a list of numbers and an empty list to store the reversed number. We then use the range()
function with a negative step value to iterate through the indices of the numbers
list in reverse order. By appending the elements from numbers to rev_numbers
using the reversed indices, we effectively reverse the order of the elements:
numbers = [1, 2, 3, 4, 5]
rev_numbers = []
for i in range(len(numbers) - 1, -1, -1):
rev_numbers.append(numbers[i])
print(rev_numbers, end=" ")
This is the output you’ll see:

Image 8 - Reversing a list in Python (image by author)
Python being Python, you can achieve the same by using a list comprehension instead of a for
loop. Take a look at the following code:
numbers = [1, 2, 3, 4, 5]
rev_numbers = [numbers[i] for i in range(len(numbers) - 1, -1, -1)]
print(rev_numbers, end=" ")
The output is identical to what we had before:

Image 9 - Reversing a list in Python (2) (image by author)
And Python being Python once again, you can use the special [::-1]
syntax to reverse a list and avoid loops or comprehensions altogether:
numbers = [1, 2, 3, 4, 5]
rev_numbers = numbers[::-1]
print(rev_numbers)
Once again, the output is identical to what we had before:

Image 10 - Reversing a list in Python (3) (image by author)
The moral of the story is that there are many ways to reverse a list in Python. The last one might not get you through a technical interview but is surely the most developer-friendly approach.
Python For Loop Range FAQ
We’ll now go over a series of commonly asked questions and answers addressing Python for loop range.
Q: Can You Iterate Through a Range in Python?
A: Absolutely! Python’s for
loop is specifically designed for iterating over sequences, including ranges. By utilizing the range()
function within a for loop, you can easily iterate through a range of numbers and perform operations on each iteration.
Just reference any code example in this article and you’ll be good to go.
Q: How Do You Start a Loop Range at 1 in Python?
A: By default, the range()
function starts from 0. However, if you want to start a loop range at 1, you can simply specify 1 as the start
argument while using the range()
function. Here’s an example:
for i in range(1, 5):
print(i, end=" ")
This is the output you will see:

Image 11 - Python range() custom starting position (image by author)
And that’s how you can start a loop range at 1 in Python, or at any value for that matter.
Q: Does range() Return a List?
A: No, the range()
function does not return a list. Instead, it returns an iterable range object. The range object is a space-efficient representation of a sequence of numbers and is optimized for performance. It generates the numbers in the range dynamically as you iterate over them, rather than generating the entire sequence upfront like a list.
However, if you need a list containing the range elements, you can convert the range object to a list using the list()
function. For example:
my_range = range(5)
my_list = list(my_range)
print(my_range, type(my_range))
print(my_list, type(my_list))
Here are the variable contents and their data types:

Image 12 - Python range() return value and data type (image by author)
Q: What Does range(len() - 1) Do in Python?
A: Using range(len() - 1)
allows you to iterate through a range of indices for a given sequence, excluding the last index. It is commonly used when you need to perform operations on all but the last element of a list, string, or other sequence types.
For example, consider the following code snippet:
nums = [1, 2, 3, 4, 5]
for i in range(len(nums) - 1):
print(nums[i], end=" ")
Here’s the output:

Image 13 - Custom length example (image by author)
Using range(len() - 1)
here allowed us to skip the last value in the list.
Summing up Python For Loop Range
In this article, we have explored the versatility and power of Python’s for
loop in combination with the range()
function. We started by understanding the different forms of the range()
function, including range(stop)
, range(start, stop)
, and range(start, stop, step)
. We then dived deep into practical examples and real-world use cases, such as reversing a list and reversing the range order, which will come in handy when working as a Python developer.
If you want to start a career in Data Science, grab your free copy of our eBook tailored to data science beginners: