Pip Install Specific Version of Package
TL;DR: You can install a specific version of a Python package using Pip by running the pip install <package_name>==<version>
command. For example, to install version 1.3.4 of Pandas, execute the pip install pandas==1.3.4
command from the Terminal.
That’s a short version and probably all you’ll ever need, but sometimes you’ll want to take more control over package installation. That’s where the rest of the article comes in.
Here’s what you’ll learn today:
Table of contents:
Why Would You Want to Install a Specific (Older) Version of a Python Package
So, why even bother with older versions of Python packages? Well, maybe you have a huge codebase that’s not compatible with the most recent package update. Maybe you’ve written the code years ago and it still works in production, but updating the package might break it. Or maybe even the most recent package version isn’t compatible with your Python version.
In other words - whatever the case may be, there are valid reasons for installing older versions of a Python package.
Let’s now go over some practical examples of how to install a specific package version with Pip.
How to Check Which Version of a Python Package is Installed
There are two useful commands you must know before proceeding to install a specific Python package version. These are:
pip show <packagename>
- Show the currently installed version of the package, its summary, author, license, dependencies, and so on.pip index versions <packagename>
- Lists all the available package versions you can install.
Let’s check them out for the pandas
Python package. The following shell command prints the currently installed version:
pip show pandas

Image 1 - Current version of the Pandas package (image by author)
It seems like Pandas version 1.5.3 is installed, and it requires numpy
, python-dateutil
, and pytz
packages to run.
But is version 1.5.3 the latest, and which other versions can we install? That’s what the next shell command answers:
pip index versions pandas

Image 2 - Available Pandas package versions (image by author)
Version 2.0.0 is the most recent, but you can go far, far back, depending on the Python version you have installed on your system or inside a virtual environment.
You now know how to check for the currently installed package version and also how to list all the available versions, so next, let’s see how to pip install a specific version of a package.
How to Install Pip Specific Version of a Python Package
There are 2 must-know ways to install a specific version of a Python package. The first one requires two commands - the first one for uninstalling the current version, and the second for installing the version you want. The second approach packs all that functionality in one shell command, so that’s the one you should use in practice.
Method 1 - Uninstall and Install
You now know which version of Pandas you have installed, and which versions you can potentially install, depending on your Python version.
In our case, we have version 1.5.3, but we want to go back to version 1.3.4. To do so, we first have to uninstall the current version:
pip uninstall pandas
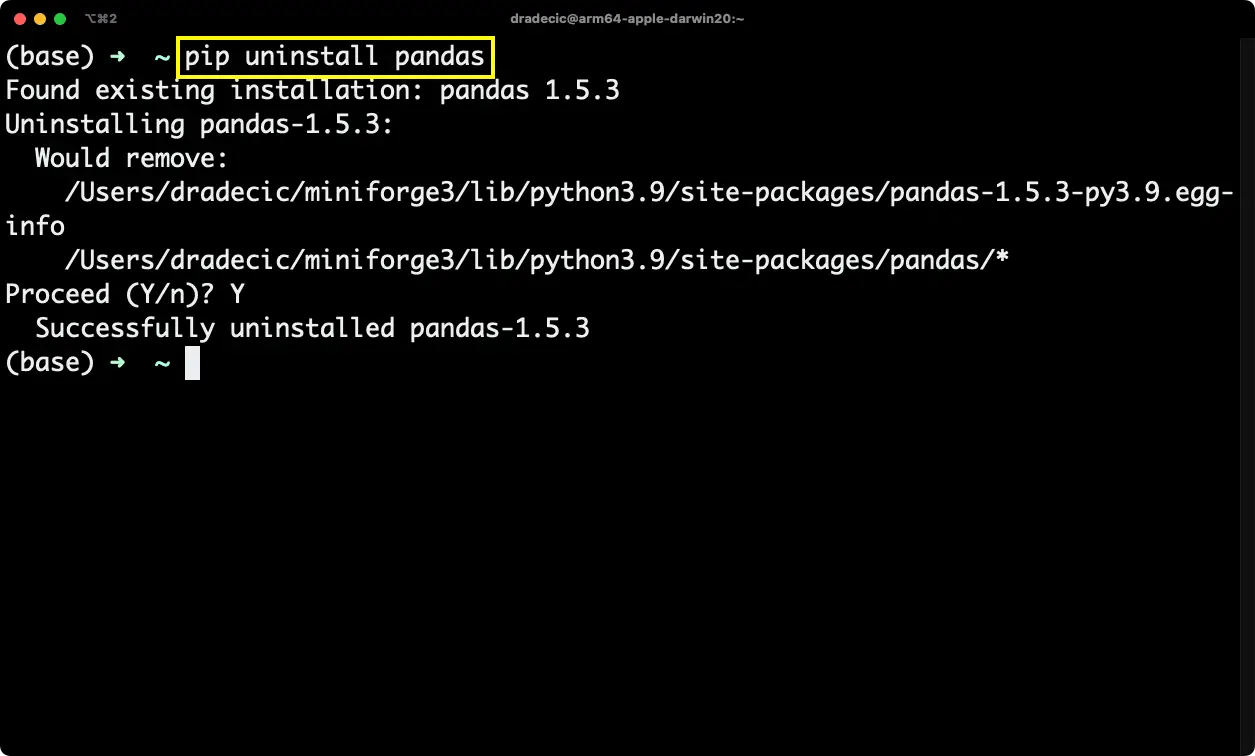
Image 3 - Uninstalling a Python package with pip (image by author)
And now, pip install specific version of Pandas by running the following command:
pip install pandas==1.3.4

Image 4 - Installing a specific version of a Python package with Pip (image by author)
It seems like everything went fine, but how can you make sure version 1.3.4 was installed? Spoiler alert: You already know the command:
pip show pandas
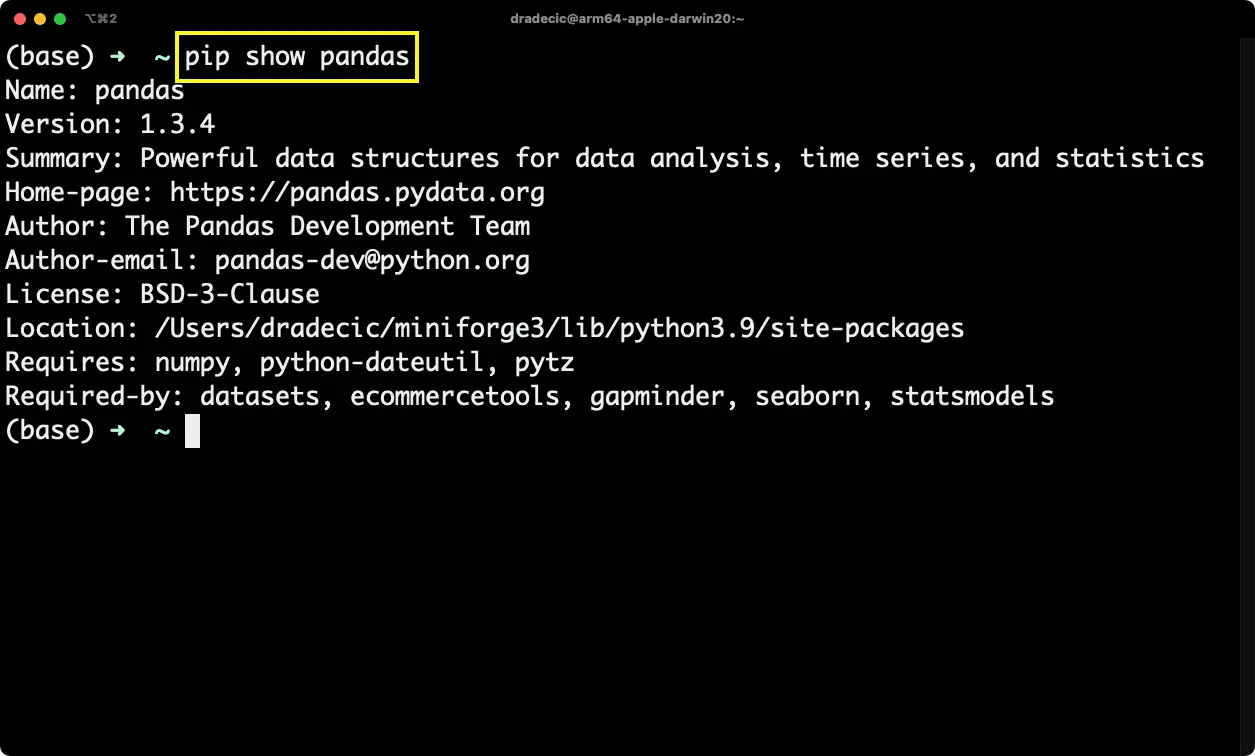
Image 5 - Currently installed Pandas version (image by author)
Pandas 1.3.4 is successfully installed on our system, so we can mark our job here as done.
Method 2 - Overwrite the Installed Package
The superior approach to the one from the previous section is to install a specific version of a Python package with a single shell command. You can do so by appending the --ignore-installed
flag when installing a specific version of the package.
Here’s an example - it installs Pandas version 2.0.0 and overwrites version 1.3.4 which was installed moments ago:
pip install pandas==2.0.0 --ignore-installed
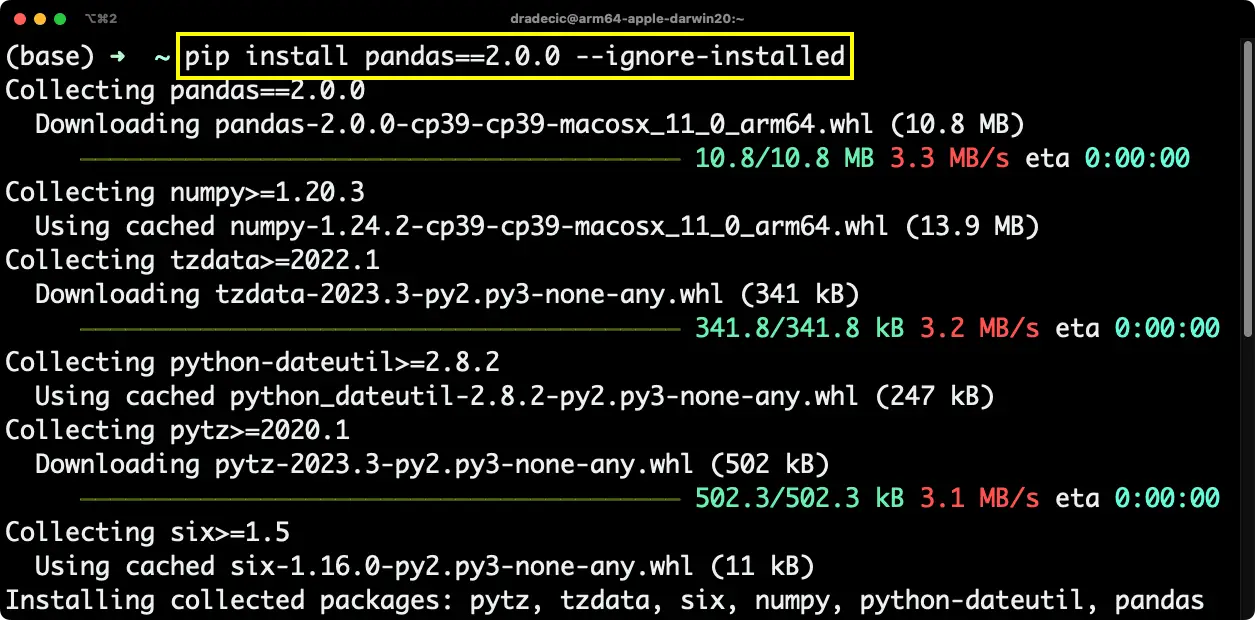
Image 6 - Overwriting the current package version (image by author)
And just to verify, you can use the pip show
command:
pip show pandas

Image 7 - Currently installed Pandas version (image by author)
And that’s two ways to pip install specific version of a Pandas package. Will it always be that easy? Probably not, so continue reading for a more production-ready use case.
How to Install Multiple Python Packages with Specific Versions
Installing Python packages one by one is fine in your local environment, but it doesn’t scale in production. That’s where you’ll typically have a requirements.txt
file which contains all Python dependencies for the project alongside their specific version.
Wait, what is a requirements.txt file? Read this article if you want to manage Python dependencies like a sane person.
The requirements.txt
is typically structured like this:
package_1==version_1
package_2==version_2
...
In short, there’s a list of Python dependencies with their respective versions. The question now is, Can you install all of them with a single command? And the answer is - yes, you can.
Here’s what the file looks like on our end:
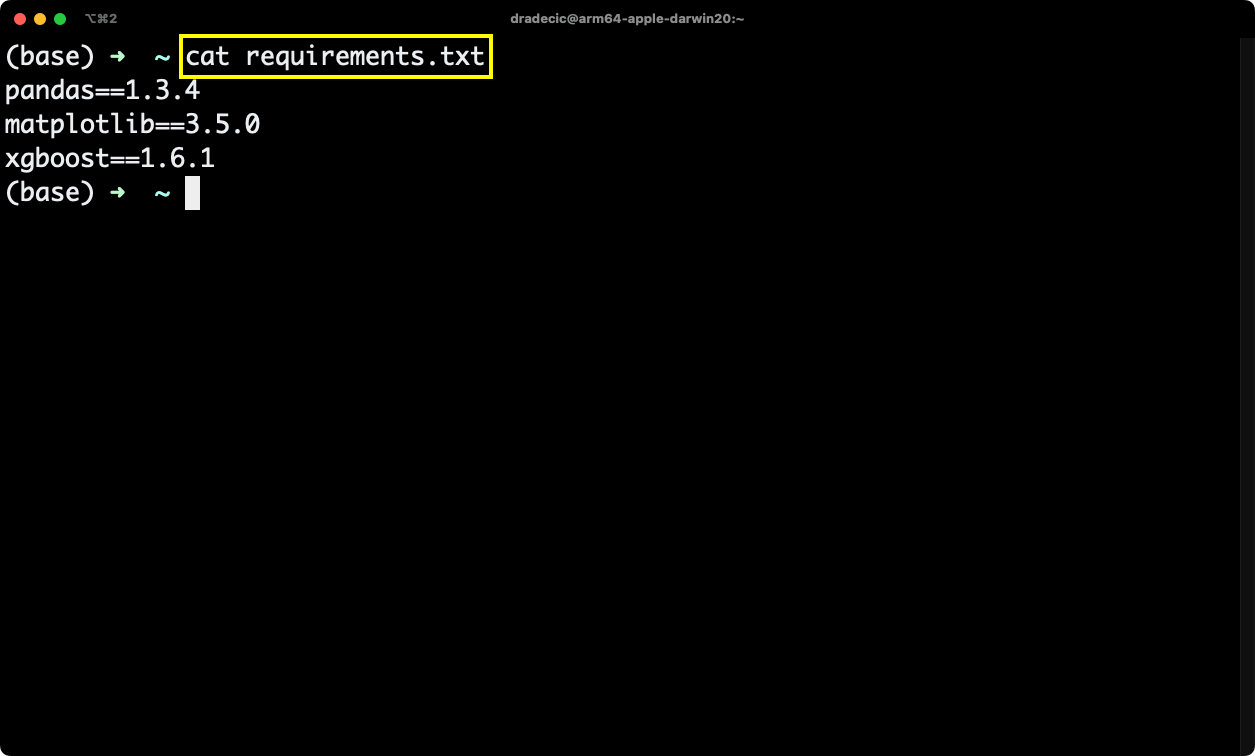
Image 8 - Contents of the requirements.txt file (image by author)
And this is the command you should run if the requirements.txt
file is in the same directory as your shell:
pip install -r requirements.txt
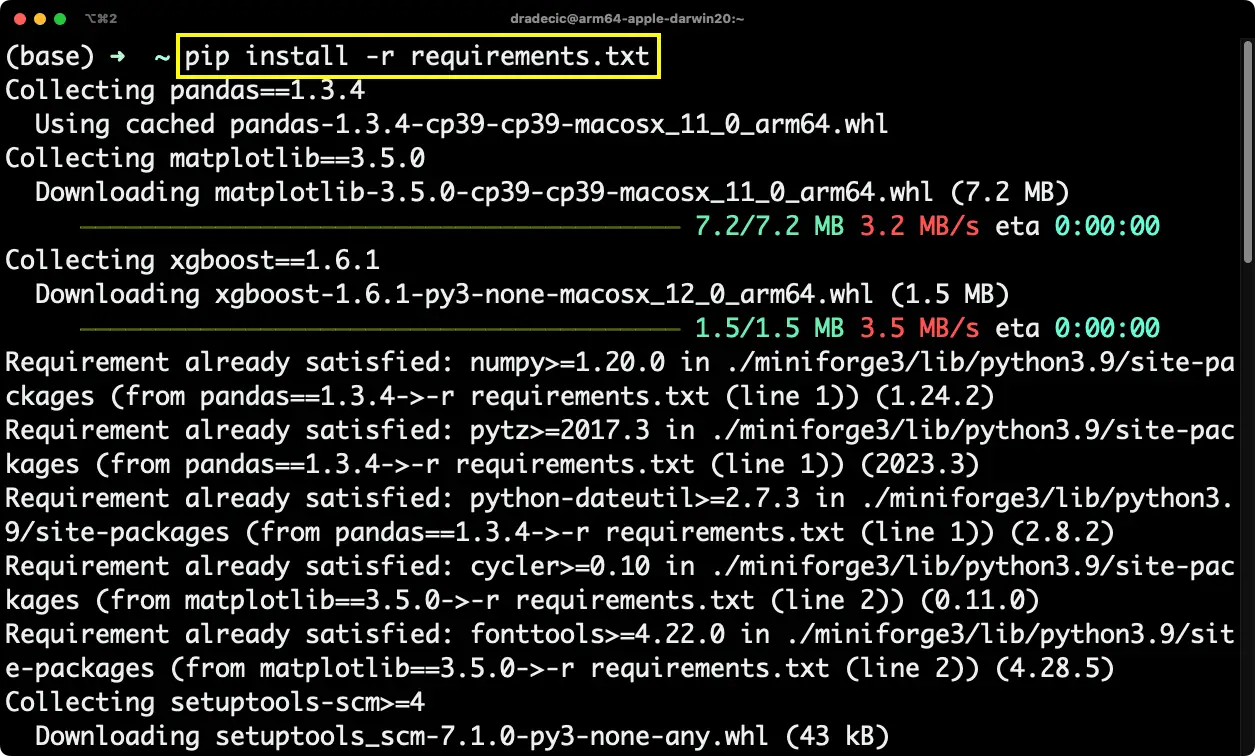
Image 9 - Installing multiple Python dependencies with a single command (image by author)
In case your requirements.txt
file is located elsewhere, simply provide an absolute or relative path after -r
. That’s it!
Now, let’s verify the correct package versions were installed:
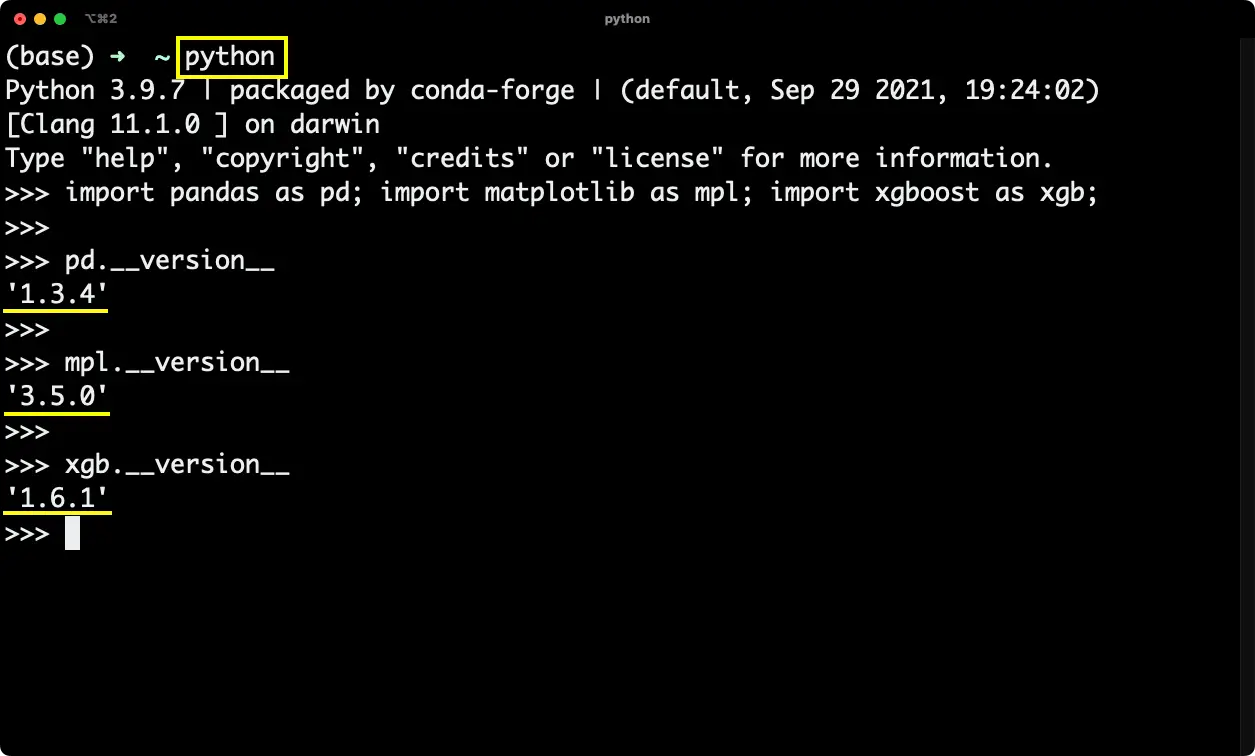
Image 10 - Checking Python package versions (image by author)
And that’s how you can install multiple Python packages at once. Let’s make a short recap next.
Summing up Pip Install Specific Version
Project dependency management doesn’t have to be difficult. You can use Python’s pipreqs module to keep track of the packages used within a project and to create a requirements.txt
file, and then apply everything learned in this article to transfer the same set of dependencies to another machine, or a production environment.
You can also pip install specific version of a Python package one by one, which is fine for a local development environment, and this article showed you a couple of ways to do so. By far better approach is to have a dedicated file for the dependencies, and install them all at once.
How do you keep track of project dependencies? Do you install a specific version of a Python package, or do you stick with the latest releases? Make sure to let me know in the comment section below.