Python Timestamp
Do you know what’s the number one thing Junior and Senior Developers have in common? They both don’t know how to work with dates without referencing the manual. It’s just one thing that’s too difficult to remember for some reason. Well, not anymore!
Python Timestamp plays a crucial role in various applications and systems, as they record the exact date and time of a particular event, thus ensuring accurate data tracking and better decision-making. In Python, timestamps can be easily generated and manipulated using the datetime
module, which enables developers to work with and manipulate date and time data effortlessly.
The datetime
module in Python provides powerful functions to handle dates, times, and time intervals. One significant aspect of this module is its ability to create timestamps, which are encoded representations of the date and time of an event, precise down to microseconds. These timestamps follow the POSIX standard and can be generated, parsed, and converted into various formats using the utilities provided by the datetime module.
In this article, we will explore the different ways to work with timestamps in Python, including generating, converting, and comparing timestamps. Throughout the discussion, we will touch upon key functions, techniques, and best practices to fully understand and effectively leverage timestamps in Python applications.
Let’s dig in!
Table of contents:
Understanding Timestamps
Timestamps are crucial elements in modern programming, especially for tasks involving time-series data or measuring the time elapsed between two events. In this section, we will explore what timestamps are, how they work with Python, and the difference between naive and aware timestamps.
Unix Timestamp
A Unix timestamp, also known as Epoch time, is the number of seconds elapsed since January 1, 1970, 00:00:00 UTC. The Unix timestamp is widely used as a standardized method to represent time based on the POSIX timestamp standard. Some of the advantages of using Unix timestamps are:
- Portability: Works with different operating systems and programming languages.
- Easy comparison: Comparing timestamps in seconds makes it simple to calculate durations and time intervals.
- Compact representation: It’s easier to store, transmit, and manipulate integers than complex date-time objects.
Python datetime Object
In Python, the datetime module provides classes to work with dates and times effortlessly. The most commonly used classes are:
date
: Represents date (year, month, and day) in the Gregorian calendar.time
: Represents time (hours, minutes, seconds, and microseconds) in a 24-hour format.datetime
: Combines both date and time features into a single object.
To work with timestamps, you can use the datetime
class’s methods, such as fromtimestamp()
and timestamp()
, to convert Unix timestamps to datetime
objects and vice versa.
These are the library imports you’ll need today, so make sure to stick them at the top of your script/notebook:
import pytz
from datetime import datetime, date, time, timedelta, timezone
Onto the first example. The following code snippet will convert a Unix timestamp to a Python datetime
object:
# Convert Unix timestamp to datetime object
unix_timestamp = 1687392000
dt_object = datetime.fromtimestamp(unix_timestamp)
print(dt_object)
Here is the output:
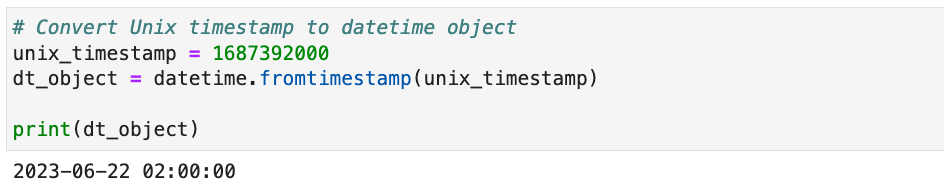
Image 1 - Unix timestamp to datetime (Image by author)
You can also go the other way around - convert a Python datetime
object into a Unix timestamp:
# Convert datetime object to Unix timestamp
current_time = datetime.now()
unix_timestamp = current_time.timestamp()
print(unix_timestamp)
This is what you will see:
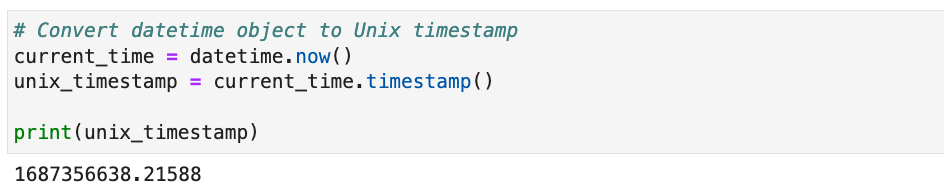
Image 2 - Datetime to Unix timestamp (Image by author)
Naive and Aware Timestamps
Python’s datetime
module distinguishes between two types of timestamps: naive and aware.
- Naive timestamps don’t include any timezone information, meaning they only record local time without considering daylight saving or other adjustments. As a result, naive timestamps can be ambiguous because they do not indicate the specific location or context in which the time was recorded.
- Aware timestamps include a timezone as part of the object, offering a more accurate representation of the actual time at a particular location. By adding timezone, you can work with precise time measurements that always account for any contextual changes in timekeeping.
To start, let’s first create a naive datetime
object:
# Naive datetime object
naive_dt = datetime.now()
print(naive_dt)
Here’s what you’ll see:

Image 3 - Datetime object (Image by author)
You can now convert into into a timezone-aware datetime
object with UTC timezone by running the following code:
# Make the datetime object timezone-aware with UTC timezone
aware_dt = naive_dt.replace(tzinfo=timezone.utc)
print(aware_dt)
This is the outut:

Image 4 - Timezone aware datetime object (Image by author)
Similarly, you can leverage the pytz
module to convert your datetime
object to a specific timezone, as shown below:
# Convert naive datetime to a specific timezone (e.g., US/Eastern) using pytz
eastern_tz = pytz.timezone("US/Eastern")
aware_dt = naive_dt.astimezone(eastern_tz)
print(aware_dt)
Here are the results:
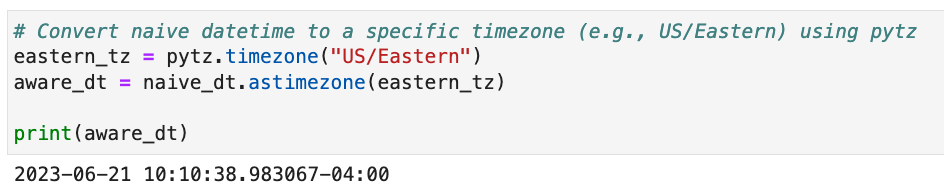
Image 5 - Datetime object in a specific timezone (Image by author)
By understanding the differences between Unix timestamps, datetime
objects, naive and aware timestamps in Python, you can manage date and time operations more effectively in your programming tasks.
Python Datetime Module - An In-Depth Guide
To work with dates and times in Python, you’ll leverage the datetime
module. You already have it imported, so that’s one less task you need to do.
Basic Datetime Functions
The datetime
module provides several classes, including date
, time
, datetime
, and timedelta
. These classes help you represent and manipulate dates, times, and intervals.
datetime.date
It represents a date (year, month, and day) in the Gregorian calendar. You can create a date object using the date(year, month, day)
constructor. To get the current date, you can use the date.today()
function:
today = date.today()
print(f"Today's date: {today}")
This is what you will see:

Image 6 - Today’s date (Image by author)
datetime.time
It represents an idealized time, storing hour, minute, second, and microsecond components. You can create a time object using the time(hour, minute, second, microsecond)
constructor:
t = time(13, 45, 30)
print(f"Time object: {t}")
Here’s the output:

Image 7 - Custom time object (Image by author)
datetime.datetime
It represents a combination of a date and a time. It has all the attributes of both date
and time
classes. You can create a datetime object using the datetime(year, month, day, hour, minute, second, microsecond)
constructor:
dt = datetime(2023, 6, 21, 17, 35, 22)
print(f"Datetime object: {dt}")
Here are the results:

Image 8 - Custom datetime object (Image by author)
datetime.timedelta
It represents a duration, as the difference between two dates or times. You can perform arithmetic operations with timedelta
objects to manipulate dates and times:
delta = timedelta(days=7)
next_week = today + delta
print(f"Next week's date: {next_week}")
This is what you’ll get printed to the console:

Image 9 - Timedelta in Python (Image by author)
Datetime Formatting in Python
The strftime()
function allows you to format a datetime
object into a string with a specific format. You can use format codes to represent components of the date and time. Here are some commonly used format codes:
%Y
- Year with century%y
- Year without century%m
- Month as a zero-padded decimal number%d
- Day of the month as a zero-padded decimal number%H
- Hour (24-hour clock) as a zero-padded decimal number%M
- Minute as a zero-padded decimal number%S
- Second as a zero-padded decimal number
Here’s an example of formatting a datetime
object using strftime()
:
dt = datetime(2023, 6, 21, 17, 35, 22)
formatted_dt = dt.strftime("%Y-%m-%d %H:%M:%S")
print(f"Formatted datetime: {formatted_dt}")
This is the output:

Image 10 - Datetime to string (Image by author)
By working with the Python datetime
module, you can easily handle dates and times in your applications. The module provides various classes and functions to work with date and time data, making it a powerful tool for developers.
Converting Timestamps in Python
In this section, we will discuss how to convert timestamps between datetime objects and Unix timestamps in Python. This is a crucial step when working with time data as it allows us to manipulate and compare time-based information more efficiently.
From Datetime to Unix Timestamp
Converting a datetime object to a Unix timestamp is a straightforward process using the .timestamp()
method. First, we need to create a datetime object, which can be achieved using the datetime.now()
function, and then use the .timestamp()
method to convert the datetime object to a Unix timestamp:
current_datetime = datetime.now()
unix_timestamp = current_datetime.timestamp()
print(unix_timestamp)
This will return the Unix timestamp as a floating-point number, representing the seconds since January 1, 1970 (the Unix epoch), as you can see from the image below:

Image 11 - Unix timestamp (Image by author)
From Unix Timestamp to Datetime
To convert a Unix timestamp to a datetime object, we can use the fromtimestamp()
method. Let’s assume we have a Unix timestamp unix_timestamp
. We can convert it to a datetime object as follows:
converted_datetime = datetime.fromtimestamp(unix_timestamp)
print(converted_datetime)
The converted_datetime
resulting from this operation will be a datetime object with the corresponding date and time information:

Image 12 - Unix timestamp to datetime (Image by author)
In summary, the conversion between datetime objects and Unix timestamps can be easily achieved using Python’s built-in datetime
module. The methods .timestamp()
and datetime.fromtimestamp()
make it simple to switch between these two formats, allowing for more efficient time-based data manipulation and comparison.
Working with Time Zones in Python
Working with time zones in Python can be achieved using the tzinfo
class from the datetime
module. This class provides an abstract base class for time zone information and is used together with the timezone
class to manipulate timezone-aware datetime
objects. The timezone
class is a subclass of tzinfo
and represents a fixed offset from the UTC (Coordinated Universal Time)
Tzinfo and Timezone Class
Creating a timezone object with a specific offset can be done like this:
offset = timedelta(hours=2)
timezone_obj = timezone(offset)
print(timezone_obj)
Here’s what you’ll see printed:

Image 13 - Timezone object (Image by author)
UTC and Time Zone Conversions
When working with dates and times, it’s essential to be aware of the different time zones and their relationship with UTC. You can use the datetime
module to create timezone-aware datetime
objects and convert between UTC and local time. The current UTC time can be obtained using the following code
current_utc = datetime.utcnow()
print(current_utc)
This is the output:

Image 14 - Current UTC time (Image by author)
To convert a naive (without timezone information) datetime
object to a timezone-aware one, you can use the replace()
method along with a timezone
object:
naive_dt = datetime.now()
aware_datetime = naive_dt.replace(tzinfo=timezone_obj)
print(aware_datetime)
Here are the results:

Image 15 - Timezone-aware time (Image by author)
Local Time and UTC Offset
Determining the local time from a given timezone-aware datetime
object is as simple as using the astimezone()
method with the desired time zone
local_datetime = aware_datetime.astimezone(timezone_obj)
print(local_datetime)
This is what you’ll see:

Image 16 - Local datetime (Image by author)
To obtain the UTC offset in seconds for a specific timezone, utilize the utcoffset()
method of the timezon
e object with a given datetime
object:
dt = datetime(2023, 6, 21, 17, 35, 22)
utc_offset = timezone_obj.utcoffset(dt)
offset_in_seconds = utc_offset.total_seconds()
print(offset_in_seconds)
Here are the results:
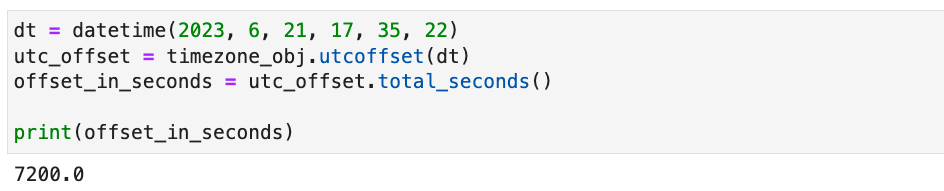
Image 17 - Timezone offset (Image by author)
Keep in mind that the UTC offset may change due to daylight saving time (DST) adjustments.
Manipulating and Formatting Dates and Times
This section will teach you how to parse Python strings into datetime
objects and how to perform some basic datetime
arithmetic.
Parsing Strings into Datetime
The Python datetime
module provides the strftime()
method to format date and time as strings. This method enables customization of the output format by using format codes.
Here’s one usage example:
date_string = "2023-06-21 14:30:59"
date_format = "%Y-%m-%d %H:%M:%S"
datetime_object = datetime.strptime(date_string, date_format)
print(f"Datetime object: {datetime_object}")
This is what you’ll see printed to the console:
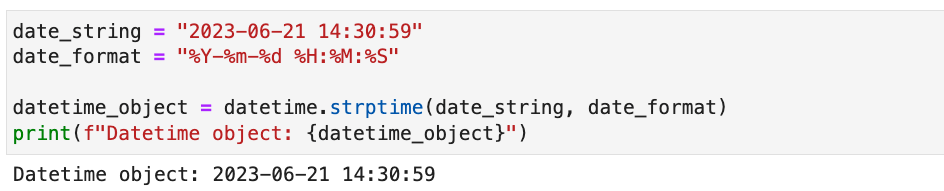
Image 18 - String to datetime (Image by author)
Datetime Arithmetic
Python’s datetime
module also supports arithmetic operations on datetime objects. To perform calculations, you can use the timedelta
class for durations.
For example, you can add durations:
now = datetime.now()
one_week = timedelta(weeks=1)
future_date = now + one_week
print(f"Future date: {future_date}")
Here are the results:
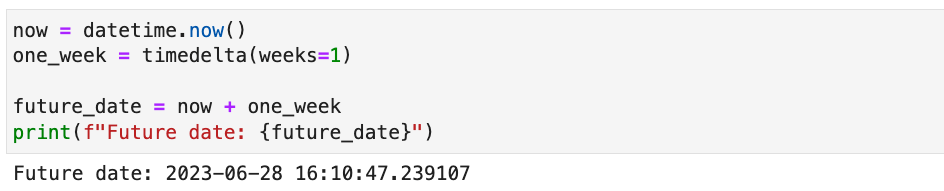
Image 19 - Adding datetime objects (Image by author)
Or you can subtract them:
past_date = now - one_week
print(f"Past date: {past_date}")
This is what you’ll see printed:

Image 20 - Subtracting datetime objects (Image by author)
Extracting Attributes
To extract specific attributes from a datetime object, you can use the following properties:
year
: the year as an integer, e.g., 2023month
: the month as an integer, e.g., 5day
: the day of the month as an integer, e.g., 21hour
: the hour (24-hour clock) as an integer, e.g., 14minute
: the minute as an integer, e.g., 30second
: the second as an integer, e.g., 59
Example of attribute extraction:
now = datetime.now()
print(f"Year: {now.year}")
print(f"Month: {now.month}")
print(f"Day: {now.day}")
print(f"Hour: {now.hour}")
print(f"Minute: {now.minute}")
print(f"Second: {now.second}")
Here are the results:
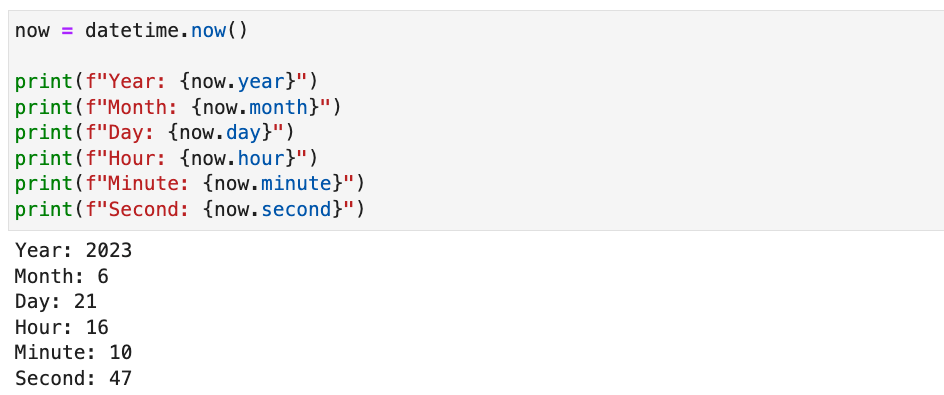
Image 21 - Extracting attributes from a datetime object (Image by author)
By utilizing the methods, classes, and properties mentioned above, you can efficiently manipulate and format dates and times in Python. Remember, the datetime
module is your go-to resource for working with dates and times, providing comprehensive functionality for common operations.
Python Timestamp - Advanced Concepts
This section will go over a couple of advanced use cases you might find intriguing.
Handling Leap Seconds
Leap seconds are occasional adjustments of one second to account for Earth’s slowing rotation. They can occur in distinct timestamps within the same second resulting in the same POSIX timestamp value.
The Python time
library handles Leap seconds as per the system’s internal configuration. However, it doesn’t have a direct method to add leap seconds in time handling. To ensure consistency and avoid issues with leap seconds, you can use the astropy.time
library.
But first, you need to install it:
pip install astropy
And now onto the usage example:
from astropy.time import Time
t = Time("2023-06-21T00:00:00", scale="utc", format="isot")
t += 1
print(f"Time after one second (including leap second): {t.isot}")
Here’s the output:
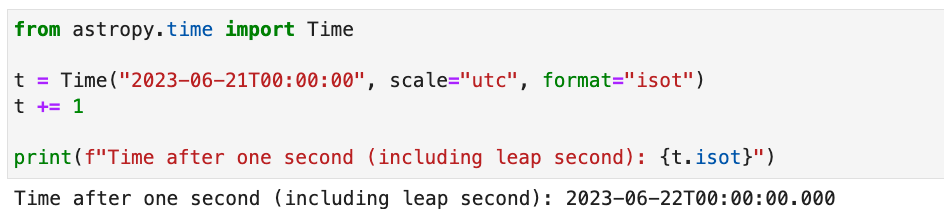
Image 22 - Leap seconds (Image by author)
This approach considers any leap seconds present according to the International Atomic Time (TAI).
Working with Timestamps in Higher Precision
Python’s built-in datetime package provides timestamps typically with a precision up to microseconds. However, you may encounter timestamps with higher precision like nanoseconds. These can be parsed using the numpy library:
import numpy as np
timestamp_str = "2023-06-21T08:17:14.078685237Z"
dt = datetime.strptime(timestamp_str[:-9], "%Y-%m-%dT%H:%M:%S.%f")
timestamp = np.datetime64(dt)
print(f"Parsed high-precision timestamp: {timestamp}")
This converts the high-precision timestamp (ISO 8601) to a numpy.datetime64
object:
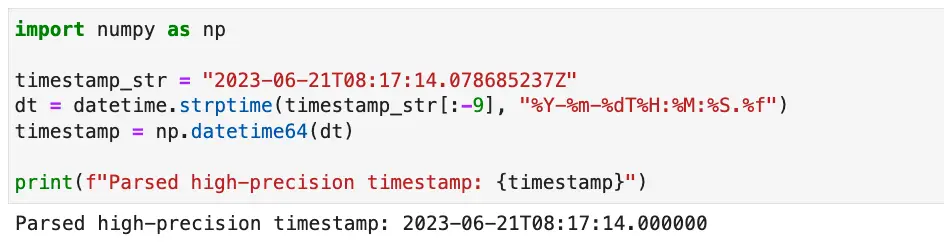
Image 23 - Higher-precision timestamp in Numpy (Image by author)
You can also represent timestamps as floating-point numbers with nanosecond precision using the pandas.Timestamp
function:
import pandas as pd
ts = pd.Timestamp(timestamp_str)
print(f"Timestamp (in ns): {ts.value}")
Here’s the output:

Image 24 - Higher-precision timestamp in Pandas (Image by author)