Python If Not
TL;DR: Python if not statement allows you to run code based on the negation of a certain condition. It’s identical to using the !
operator in other programming languages, such as Java.
Python is one of the most popular and beginner-friendly programming languages out there - and for a good reason. When written correctly, it can read as plain English. That’s especially the case with the if not
keyword, and you’ll learn all about it today. Long story short, it allows you to execute code based on the negation of a certain condition.
The if not
syntax in Python is a combination of the if
conditional statement and the not
logical operator. The if
statement is used to evaluate whether a condition is true or false, while the not
keyword reverses the result of the condition, allowing for inverse testing. This powerful duo provides developers with the ability to perform actions when a specific condition is not met, thereby adding more flexibility and control to their code.
As always, the best way to learn about it is by seeing a bunch of hands-on examples, so let’s dive in!
Table of contents:
Introduction to Python If Not Statement
The Python if not
statement is a powerful tool for controlling the flow of your program. It is often used to test whether a certain condition is false or to reverse the result of a conditional expression. In this section, we will explore the syntax and examples of using the if not
statement in Python.
Syntax
The basic syntax of an if not
statement in Python is as follows:
if not condition:
# code to execute when the condition is False
Here, the if not
statement checks if the given condition
is False, and if so, it executes the code within the indented block. The condition
can be a boolean value, expression, or even an object. Note that the not
keyword is a logical operator that reverses the result of the given condition.
Examples
Here are some examples of common use cases for the if not
statement in Python:
1. Checking if a variable is None
:
var = None
if not var:
print("Variable is None.")
This is the output you should see:
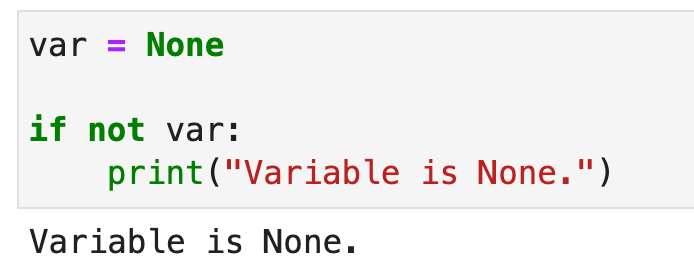
Image 1 - Checking if variable is None (Image by author)
2. Testing if a list is empty:
my_list = []
if not my_list:
print("List is empty.")
This is the output you should see:
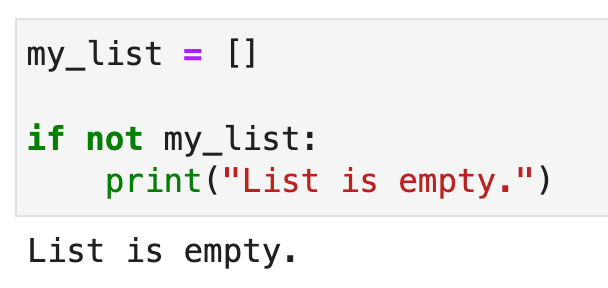
Image 2 - Testing if list is empty (Image by author)
3. Reversing a conditional expression:
a = 5
b = 10
if not a > b:
print("a is NOT greater than b.")
This is the output you should see:
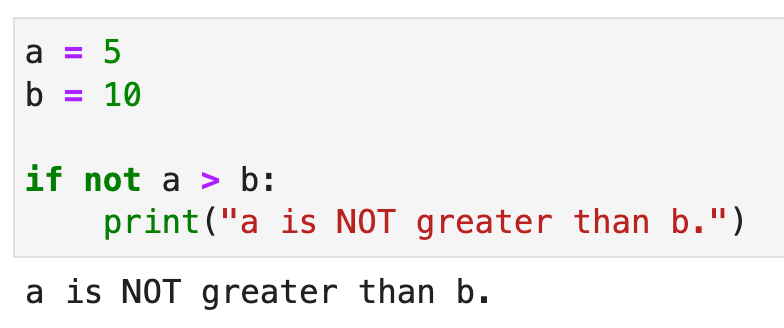
Image 3 - Reversing a conditional expression (Image by author)
4. Using if not with multiple conditions:
x = 7
if not (x > 10 or x < 2):
print("x is between 2 and 10.")
This is the output you should see:
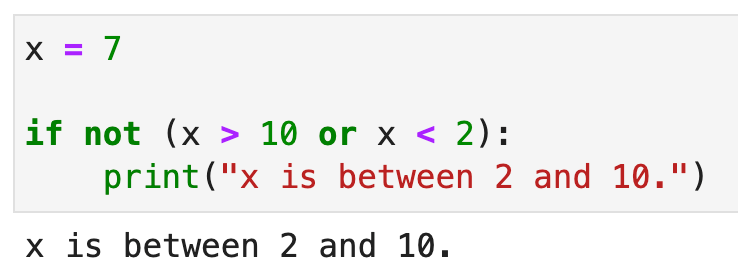
Image 4 - If not with multiple conditions (Image by author)
In each of these examples, the if not
statement is effectively used to test opposite or negative conditions, allowing you to make your code more readable and efficient. It’s an essential tool for any Python programmer, so be sure to familiarize yourself with how it works and when to use it.
Python Logical Operators in Depth
In Python, logical operators are used to evaluate expressions involving boolean values, such as True and False. These operators include not
, and
, and or
. They are essential in making complex decisions based on the outcome of multiple expressions. Let’s investigate each logical operator in more detail.
Not Operator
The not
operator is a unary operator that takes a single operand and reverses its truth value. If the input is True, the not
operator returns False - and vice versa. It’s useful when you want to check the opposite condition of an expression. Here’s an example:
is_raining = False
if not is_raining:
print("It's a sunny day!")
Here’s the output you will see:
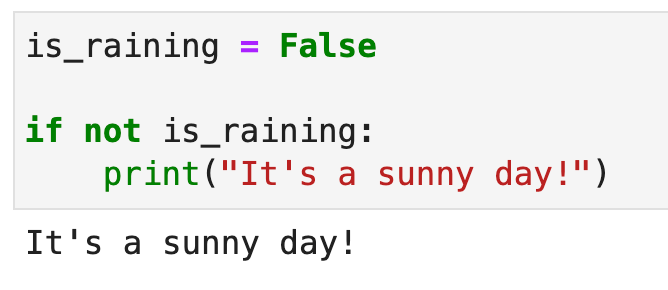
Image 5 - Not operator (Image by author)
The if not
statement checks if the is_raining variable is False. If that’s the case, it prints a message indicating it’s a sunny day.
And Operator
The and
operator takes two operands and evaluates to True only if both of the operands are true. If one or both of the operands are False, the and operator returns False. It’s helpful in situations where you want to perform an action when multiple conditions are satisfied. Here’s an example:
temperature = 75
is_sunny = True
if temperature > 70 and is_sunny:
print("It's the perfect day for outdoor activities!")
This is what you should see printed out:
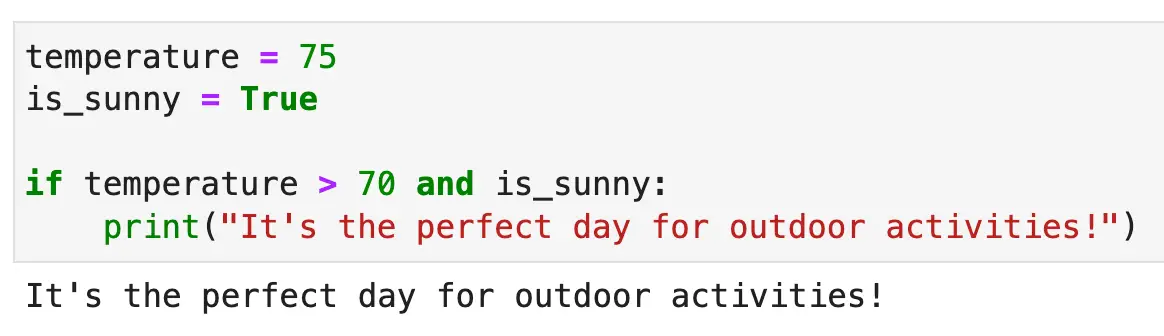
Image 6 - And operator (Image by author)
In the above example, the if
statement checks if the temperature is above 70 degrees and if it’s sunny. If both conditions are met, the message gets printed.
Or Operator
The or
operator takes two operands and evaluates to True if at least one of the operands is true. If both of the operands are False, the or operator returns False. It’s useful when you want to do something based on at least one of the conditions being met. Here’s an example:
is_weekend = True
is_holiday = False
if is_weekend or is_holiday:
print("It's time to relax!")
You’ll see the following printed to the console:
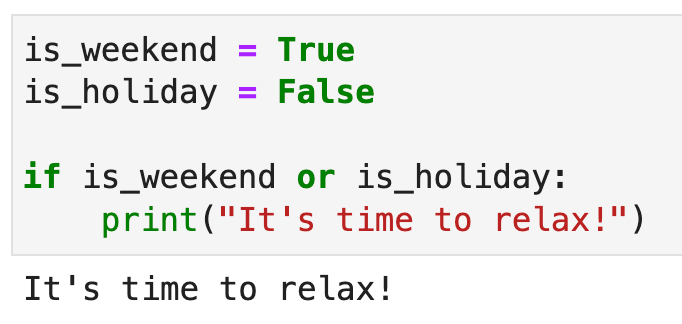
Image 7 - Or operator (Image by author)
In this example, the if
statement checks if it’s either the weekend or a holiday. If at least one of these conditions is true, the message gets printed.
These logical operators - not
, and
, and
or - play a critical role when working with boolean expressions in Python. Using them effectively allows you to create more nuanced and powerful conditional statements.
Python Conditional Statements in Depth
Conditional statements in Python allow you to control the flow of your program based on specific conditions, like if, elif, else statements. These statements are used to test for particular conditions and execute the appropriate block of code when the condition is met.
Want to implement conditional statements in one line of code? This is the article for you.
If Condition
The if
condition is used when you need to execute a specific block of code when a condition is true or met. In Python, the condition is an expression that can be evaluated as a boolean (True or False). Here’s an example:
a = 10
b = 5
if a > b:
print("a is greater than b")
Here’s the output you’ll see:
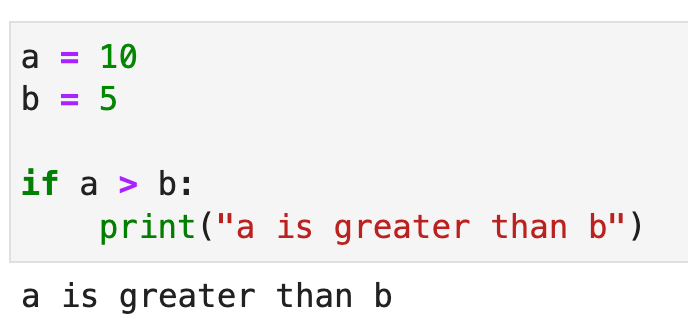
Image 8 - If statement (Image by author)
In this case, since a
is greater than b
, the print statement will be executed. Conditions can be any expression that evaluates to boolean, like checking equality (==), inequality (!=), or other logical expressions.
Elif
The elif
statement allows you to add multiple conditions to your code. It stands for “else if,” and is used when you want to check for another condition if the previous if
or elif
condition is not met. Here’s an example:
temperature = 60
if temperature > 80:
print("It's hot outside")
elif temperature < 60:
print("It's cold outside")
else:
print("The weather is pleasant")
This is what you will see:
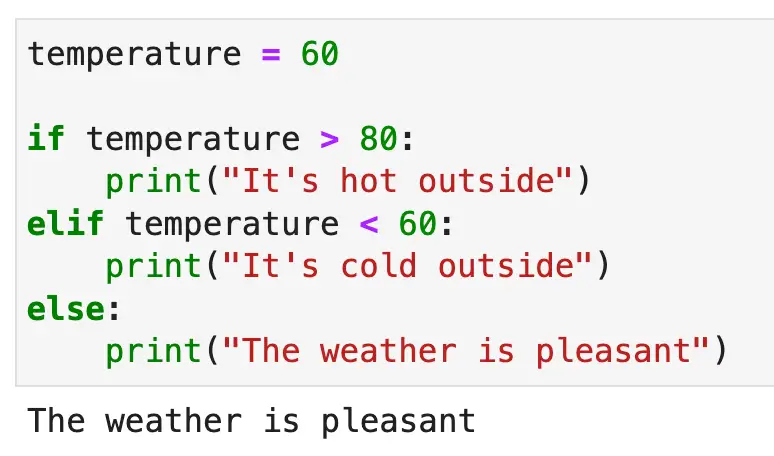
Image 9 - Elif statement (Image by author)
In this case, the program checks if temperature
is greater than 80. Because it’s not, it moves to the elif
statement and checks if temperature
is less than 60. Since neither condition is met, the else
block is executed.
Else
When using conditional statements, you may also want to include an else
block, which will execute if none of the preceding conditions are met. It acts as a catch-all for cases that don’t fit any of the specified conditions. The else
statement should be placed after all the if
and elif
statements. Here’s an example:
age = 19
if age < 13:
print("You are a child")
elif age < 18:
print("You are a teenager")
else:
print("You are an adult")
Here are the results:
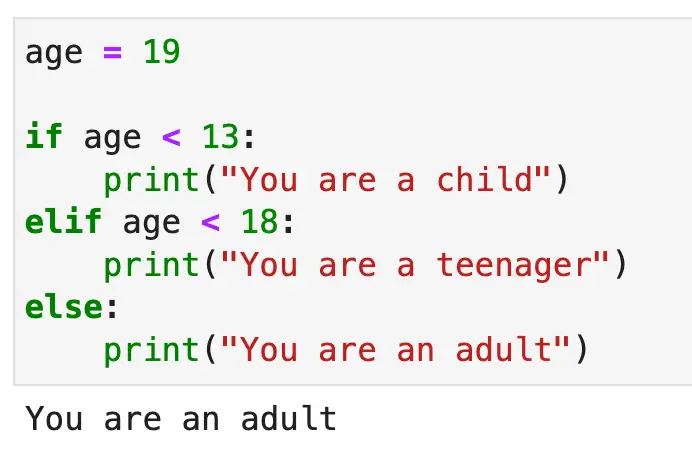
Image 10 - Else statement (Image by author)
In this case, since age
is not less than 13 and not less than 18, the else
block is executed, and “You are an adult” is printed.
Using if
, elif
, and else
statements, you can build complex conditional statements in Python to control the flow of your programs based on specific conditions, making your code more flexible and dynamic.
Python If Not on Variables
In Python, variables are used to store and manipulate data. This section focuses on working with different types of variables and how to use the if not
statement in particular contexts.
Booleans
A boolean variable represents one of two values: True or False. These are the basic building blocks for conditional expressions in Python. In combination with the if not
statement, you can create powerful conditions.
is_raining = False
if not is_raining:
print("You can go outside without an umbrella.")
This is what you will see:
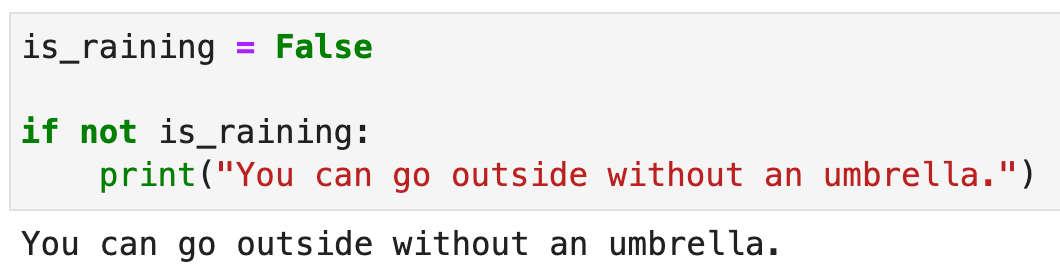
Image 11 - If not on booleans (Image by author)
The if not
statement checks if the condition is False, and if so, executes the code block within its scope. In this example, the code block will be executed only if is_raining
is False.
Numbers
Python supports different types of numeric variables, such as integers and floats. You can use numeric variables in conditional statements to check for equality, inequality, or other relationships.
x = 5
y = 10
if not x > y:
print("x is less than or equal to y.")
Here are the results:
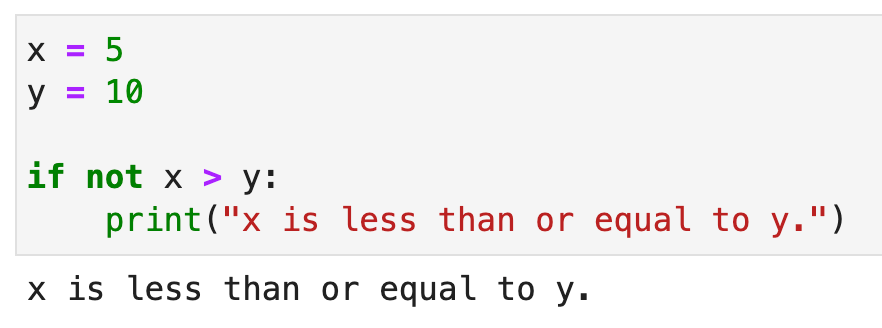
Image 12 - If not on numbers (Image by author)
The if not
statement is useful for inverting such comparisons. As in the example above, the code will execute if x is not greater than y, which means x is either less than or equal to y.
Strings
Strings in Python are sequences of characters enclosed between single or double quotes. The if not
statement can be applied to strings for checking if a string is empty or if a substring is not present.
text = "Hello, World!"
if not "Python" in text:
print("No mention of Python in the text.")
Here is the output:
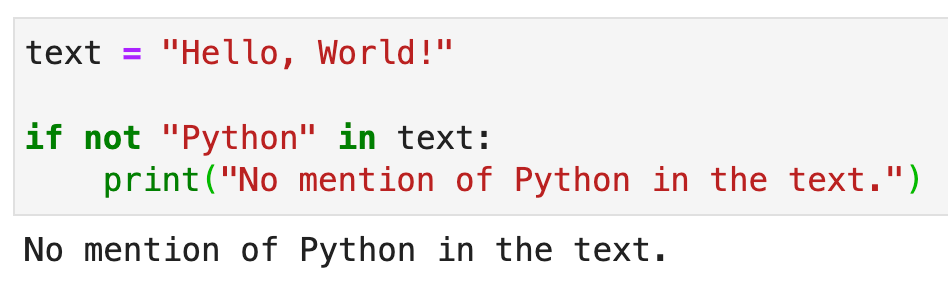
Image 13 - If not on strings (Image by author)
Here, the if not
statement is checking if the substring “Python” is not present in the text
variable. If the condition is met, the code block within its scope will execute.
When working with variables, it’s crucial to consider their data type, whether it’s a boolean value, a number (integer or float), or a string. Leveraging the if not
statement in conjunction with these data types enables powerful, conditional execution of code.
Falsy Valus and None in Python
In Python, certain values are considered “falsy” because they evaluate to False in a boolean context. Understanding the difference between falsy values and None
is important when working with conditional statements.
Falsy Objects
Falsy values in Python include:
- False
- None
- Numeric types: 0, 0.0, 0j
- Empty sequences: ‘’, [], ()
- Empty mappings: {}
When these values are put in a boolean context, such as an if
statement, they will be interpreted as False. For example:
empty_list = []
if empty_list:
print("This will not be printed")
In this example, because empty_list
is empty, the if
condition evaluates to False, and the print statement does not execute.
None Usage
None
is a special object in Python, representing the absence of a value or a null value. It is used to initialize variables that might be assigned a value later or to represent default values for optional function arguments. When comparing a variable to None
, it is recommended to use the is
keyword rather than ==
:
x = None
if x is None:
print("x is None")
Here is the output:
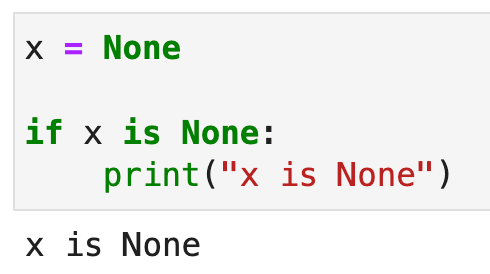
Image 14 - None in Python (Image by author)
When working with Python, it’s important to understand the distinction between falsy values and None
. Falsy values evaluate to False in boolean contexts, while None
is a special object representing the absence of a value.
Using Python If Not with Container Objects
In this section, we will explore how to use the if not
conditional statement with different types of containers in Python, such as lists, sets, tuples, and dictionaries. We’ll look into how to perform membership tests and work with empty containers.
Lists
A list in Python is an ordered collection of items. The in operator can be used to perform a membership test, checking if an item is present in a list. For instance:
my_list = [1, 2, 3, 4]
if not 5 in my_list:
print("5 is not in the list")
Here is the output:
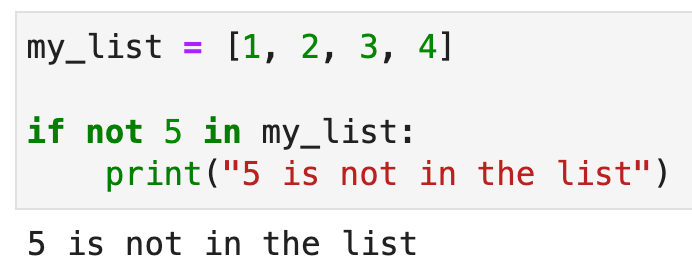
Image 15 - If not with lists (1) (Image by author)
To check for an empty list, use the not keyword in the following manner:
empty_list = []
if not empty_list:
print("The list is empty")
These are the results:
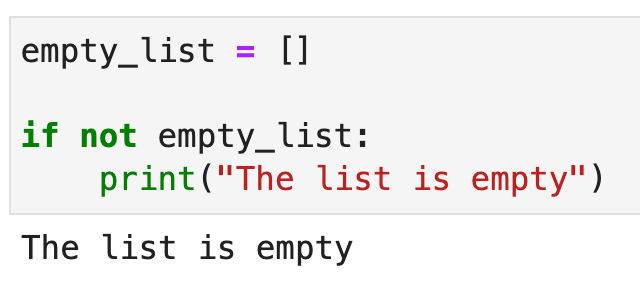
Image 16 - If not with lists (2) (Image by author)
Sets
A set is an unordered collection of unique items. In Python, you can use the in operator to test for membership in a set.
Learn more about Python sets:
Consider the following example:
my_set = {1, 2, 3, 4}
if not 5 in my_set:
print("5 is not in the set")
This is what you will see:
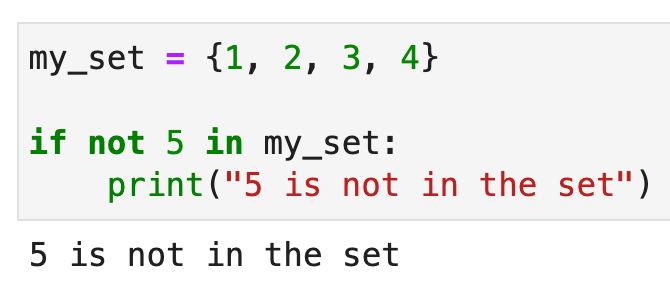
Image 17 - If not with sets (1) (Image by author)
To verify if a set is empty, simply use the not
keyword:
empty_set = set()
if not empty_set:
print("The set is empty")
Here is what you will see:
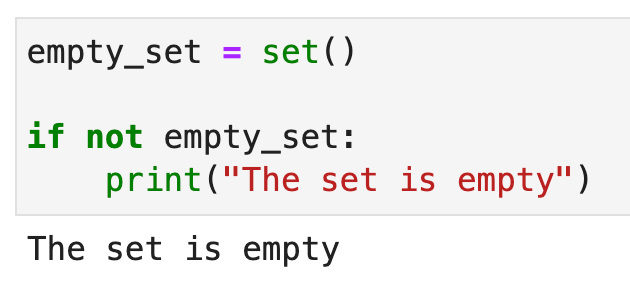
Image 18 - If not with sets (2) (Image by author)
Tuples
Tuples are similar to lists but they are immutable, meaning their elements cannot be changed after they are created. To perform a membership test, use the in
operator, as in:
my_tuple = (1, 2, 3, 4)
if not 5 in my_tuple:
print("5 is not in the tuple")
These are the results:
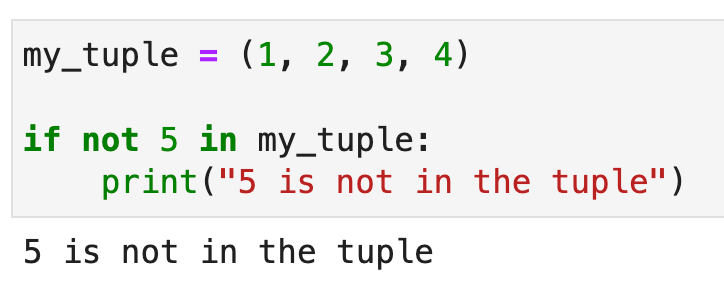
Image 19 - If not with tuples (1) (Image by author)
Checking for an empty tuple can be done using the not
keyword:
empty_tuple = ()
if not empty_tuple:
print("The tuple is empty")
Here’s what you will see:
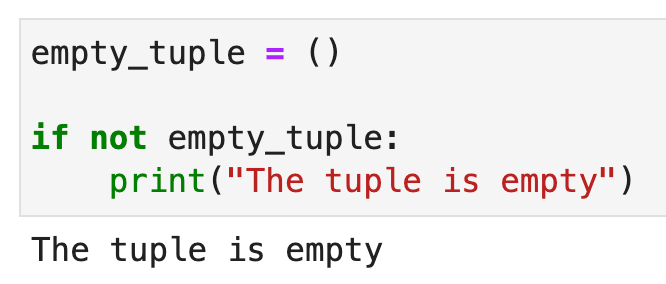
Image 20 - If not with tuples (2) (Image by author)
Dictionaries
A dictionary is a collection of key-value pairs. But what about nested dictionaries? Here’s a detailed guide..
Testing for the presence of a key in a dictionary can be done using the in
operator:
my_dict = {"a": 1, "b": 2, "c": 3, "d": 4}
if not "e" in my_dict:
print("The key 'e' is not in the dictionary")
These is the output:
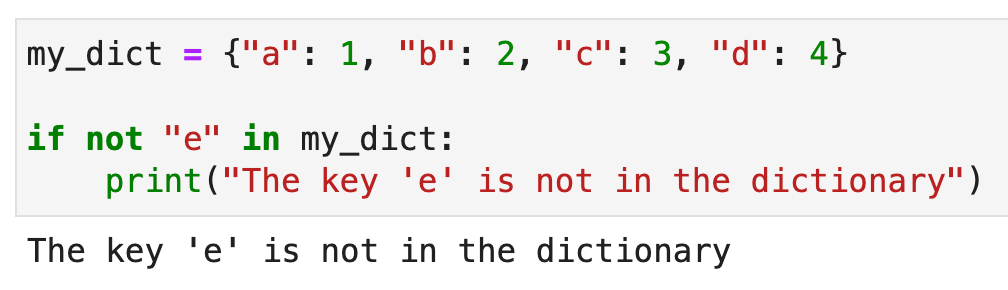
Image 21 - If not with dictionaries (1) (Image by author)
To check if a dictionary is empty, use the not keyword:
empty_dict = {}
if not empty_dict:
print("The dictionary is empty")
You will see this printed to the console:
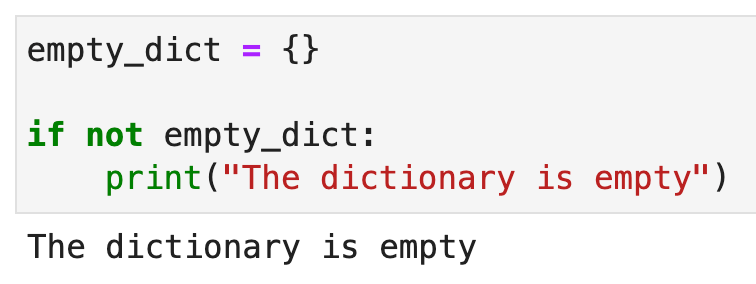
Image 22 - If not with dictionaries (2) (Image by author)
In this section, we have explored how to use if not
with different types of containers in Python, including lists, sets, tuples, and dictionaries. We have covered membership tests with the in
operator and handling empty containers using the not
keyword.
Python If Not Advanced Examples
In this section, we will cover some examples and use cases of using if not in Python programming, including validating input, checking file existence, and controlling output.
Validating Input
When processing user input, it is important to ensure the input meets certain criteria. Here’s an example of using if not
to validate user input before proceeding:
user_input = input("Enter a non-empty string: ")
if not user_input:
print("Invalid input. Please try again.")
else:
print("You entered:", user_input)
This is the result:
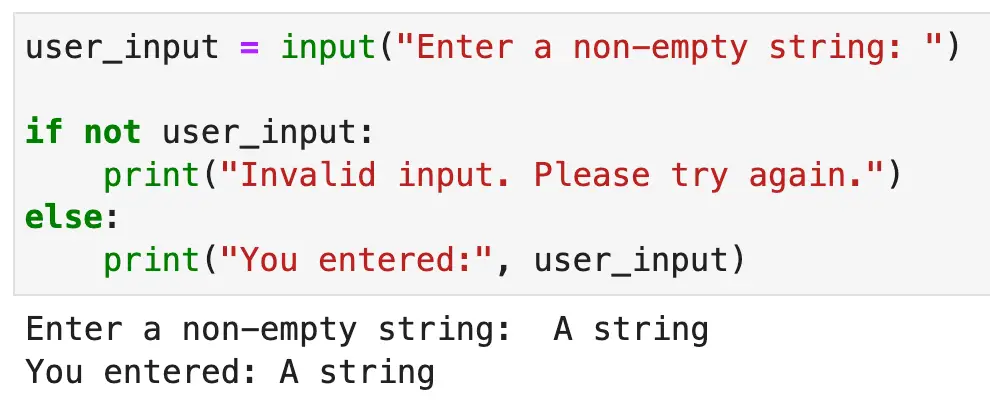
Image 23 - If not validating input (Image by author)
In this example, the code checks if the user input is empty and displays an error message accordingly.
Checking File Existence
It is common to perform file operations in Python, such as opening or deleting files. To avoid errors, it’s necessary to check if the file exists before performing an action.
import os
file_path = "example.txt"
if not os.path.exists(file_path):
print("File does not exist. Creating a new file.")
with open(file_path, "w") as new_file:
new_file.write("This is a new file!")
else:
print("File exists. Proceeding with the operation.")
Here’s what you will see:
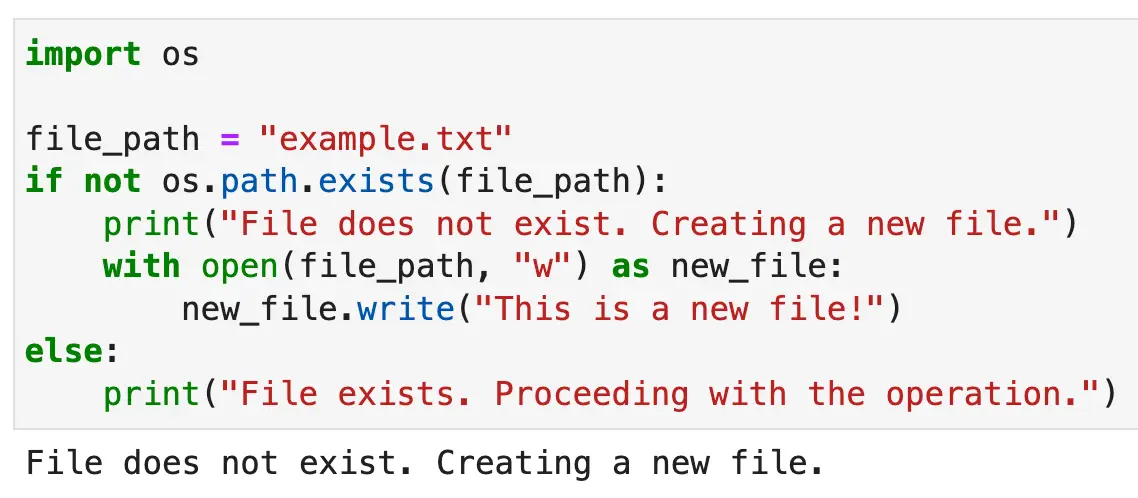
Image 24 - If not checking file existence (Image by author)
This code snippet checks if the file exists, creates a new file if it doesn’t, and proceeds with other operations if the file is found.
Controling Output
Conditional statements, including if not
, can be used to control the output shown to the user based on specific conditions. For example:
grades = {"Alice": 95, "Bob": 85, "Charlie": 75}
for student, grade in grades.items():
if not grade >= 90:
continue
print(student, "achieved an A grade.")
This is the output:
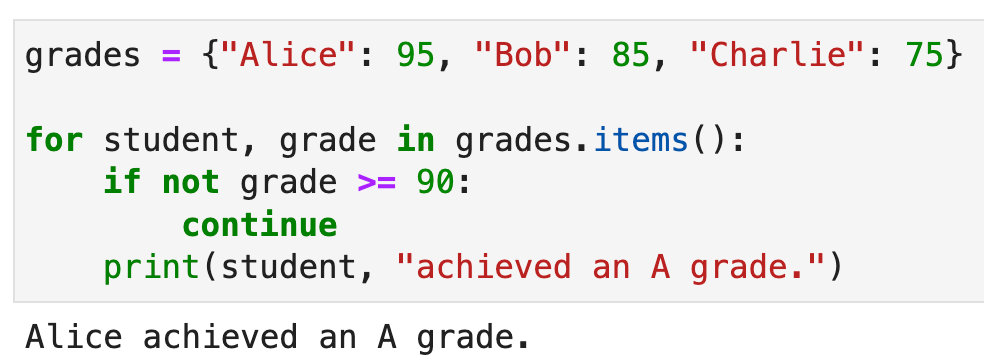
Image 25 - If not controlling output (Image by author)
In this scenario, only the names of students who have at least 90 points are displayed. By using the if not
statement, we can filter the output effectively.
These examples showcase the versatility and utility of if not
in Python programming. It provides an efficient and clear way to handle various conditions and scenarios that might arise while coding.