Pandas DataFrame to List
If you want to convert DataFrame to list in Python, you have 5 options. You can convert a single row or column to list with the tolist()
function, you can convert the entire DataFrame to a nested list, also with the tolist()
function, and you can even convert the DataFrame into a list of tuples (to_records()
function) or dictionaries (to_dict()
function).
This article will explain each of those 5 methods in detail.
Want to go the other way around? Here’s how to convert List to a Pandas DataFrame.
Regarding library imports, you’ll only need Pandas, so stick this line at the top of your Python script or notebook:
import pandas as pd
And for the data, we’ll use a made-up Pandas DataFrame of four employees described by three features - first name, last name, and email:
data = pd.DataFrame({
"First Name": ["Bob", "Mark", "Jane", "Patrick"],
"Last Name": ["Doe", "Markson", "Swift", "Johnson"],
"Email": ["[email protected]", "[email protected]", "[email protected]", "[email protected]"]
})
data
This is what the DataFrame looks like:
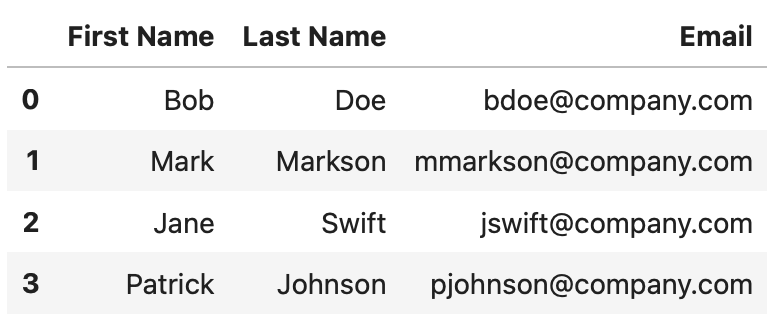
Image 1 - Starting dataset (Image by author)
Let’s get started with the first way of converting a Pandas DataFrame to a Python list.
Table of contents:
Convert Pandas DataFrame to List
You have many options if you want to convert DataFrame to list, but the process isn’t as straightforward as you might think. You can’t use a df to list function, since a Pandas DataFrame can’t be converted directly into a list.
Let’s explore what we mean by that.
Can You Convert Entire Pandas DataFrame to List?
So, what would happen if you call the tolist()
function directly on an entire Pandas DataFrame? Let’s try it out:
data.tolist()
It raises an exception since the tolist()
method isn’t available for the DataFrame object:
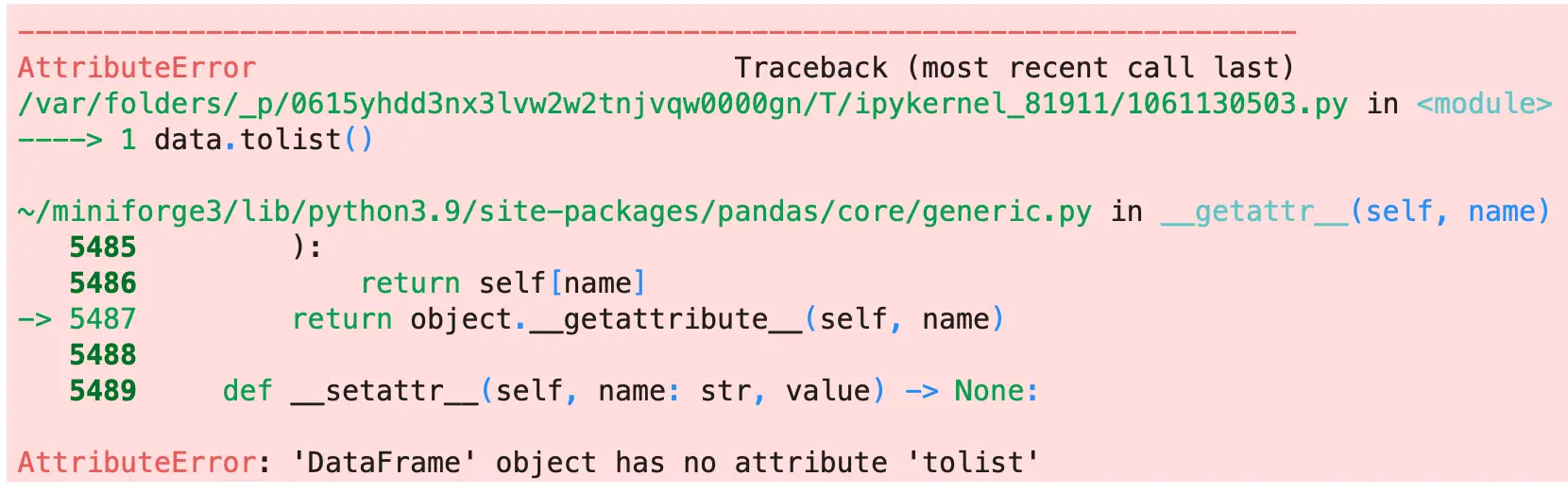
Image 2 - Exception when trying to convert an entire DataFrame to list (Image by author)
What gives? How can we make the conversion happen? The answer is easier than you might think.
How to Convert Pandas DataFrame to a Python List in a Loop
Pandas DataFrame is actually a list of lists, which means you’ll have to convert DataFrame to a nested list instead of a 1D list.
You can do so by iterating over DataFrame columns in a loop, and calling tolist()
on every column, as shown below:
out = []
for col in data.columns:
out.append(data[col].tolist())
Or, you can be a bit more Pythonic and do everything in a single line of code with list comprehensions:
[data[col].tolist() for col in data.columns]
Whatever approach you choose, this is the list you’ll end up with:
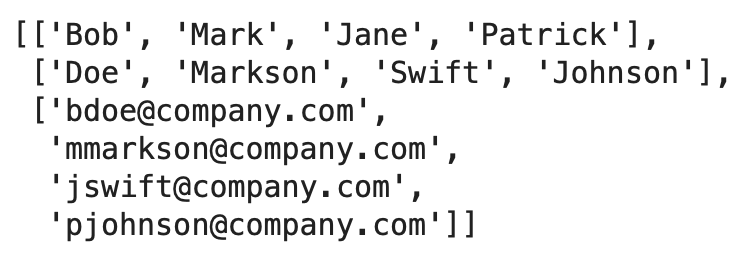
Image 3 - Pandas DataFrame to list with a loop (Image by author)
It’s a multidimensional Python list containing DataFrame columns as child list elements. The orientation is a bit messed up since one child element doesn’t represent one DataFrame record.
In practice, it’s not recommended to use this approach. Instead, you should convert a DataFrame to a Numpy array first. How? That’s what we’ll answer next.
How to Use the tolist() Function on DataFrame Values
You can access the values
property of a Pandas DataFrame, and it will return you a 2-dimensional Numpy array containing data records. Then, you can call the tolist()
function to convert everything into a Python list.
It sounds like a lot, but the syntax can’t be any shorter:
data.values.tolist()
This is the resulting list:

Image 4 - Pandas DataFrame to a list with tolist() function (Image by author)
We now have child lists oriented as records, meaning each child list represents one employee and not one column. That’s exactly what you want 95% of the time.
Convert Pandas Column to List
Sometimes, you only need to convert single Pandas column to list, and that’s what this section will go over.
You can simply select the column you need via the dataframe["column name"]
syntax, and call tolist()
on it.
Here’s an example:
data = pd.DataFrame({
"First Name": ["Bob", "Mark", "Jane", "Patrick"],
"Last Name": ["Doe", "Markson", "Swift", "Johnson"],
"Email": ["[email protected]", "[email protected]", "[email protected]", "[email protected]"]
})
data["First Name"].tolist()
The resulting Python list is 1-dimensional now, and contains first names only:

Image 5 - Converting a single column to list (Image by author)
Up next, let’s see how to convert a single row into a list.
Convert Pandas Row to List
There are times when you only need to convert a single row/record to a Python list. In our case, a single row represents information on a single employee.
To access a single row of a Pandas DataFrame, you can use the loc[]
indexer, and pass in the row number, and then convert the entire thing to a Python list. Here’s an example of how to convert the second row (index position 1) to a list:
data = pd.DataFrame({
"First Name": ["Bob", "Mark", "Jane", "Patrick"],
"Last Name": ["Doe", "Markson", "Swift", "Johnson"],
"Email": ["[email protected]", "[email protected]", "[email protected]", "[email protected]"]
})
data.loc[1].tolist()
As expected, we get a 1-dimensional list back:

Image 6 - Converting a single row to list (Image by author)
That does it for converting a single column/row to a Python list. But what about more advanced use cases? Let’s go over dictionaries and tuples next.
Convert Pandas DataFrame to a List of Dictionaries
You can convert a Pandas DataFrame to a list of dictionaries easily via the to_dict()
function call on the entire DataFrame. This will result in a conversion from a Pandas DataFrame to a list of objects.
Want to go from a Python Dictionary to a Pandas DataFrame? Here are 5 ways to do so.
To get a list of dictionaries, where each dictionary represents a single DataFrame row, you have to call the to_dict()
function and pass in an argument of orient="records"
. Here’s how:
data = pd.DataFrame({
"First Name": ["Bob", "Mark", "Jane", "Patrick"],
"Last Name": ["Doe", "Markson", "Swift", "Johnson"],
"Email": ["[email protected]", "[email protected]", "[email protected]", "[email protected]"]
})
data.to_dict(orient="records")
And this is the resulting list:
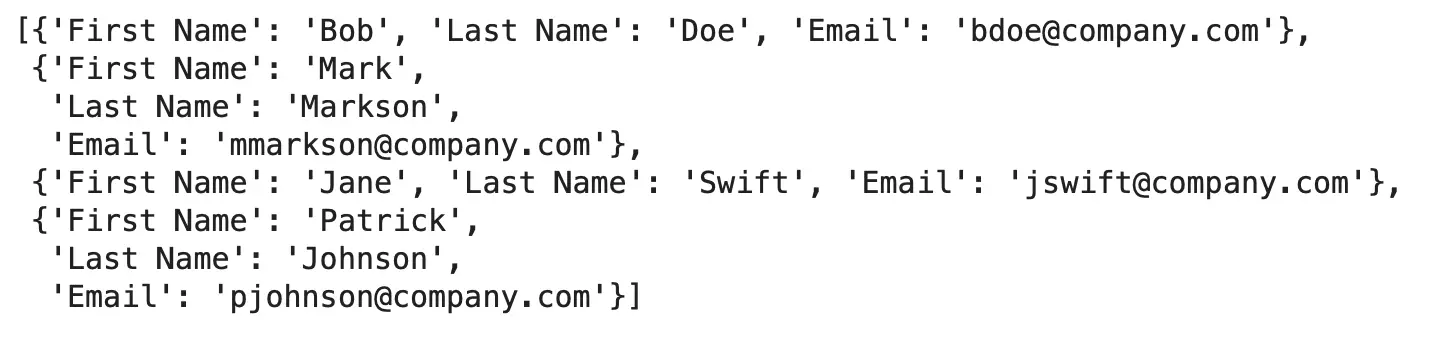
Image 7 - Pandas DataFrame to a list of dictionaries (Image by author)
Up next, let’s cover tuples.
Convert Pandas DataFrame to a List of Tuples
Think of a tuple data structure as a neat way to store multiple items in a single variable. Unlike lists, tuples are ordered and unchangeable, meaning you can’t append an item to it - you’ll have to reassign the entire thing instead.
A conversion from a Pandas DataFrame to a list of tuples boils down to calling the to_records()
function on the entire DataFrame and then chaining the tolist()
function to it.
Here’s an example in code:
data = pd.DataFrame({
"First Name": ["Bob", "Mark", "Jane", "Patrick"],
"Last Name": ["Doe", "Markson", "Swift", "Johnson"],
"Email": ["[email protected]", "[email protected]", "[email protected]", "[email protected]"]
})
data.to_records().tolist()
And here’s the resulting list of tuples;

Image 8 - Pandas DataFrame to a list of tuples (Image by author)
As you can see, tuples also contain index information. It isn’t particularly useful in our case, since we’re using a default range index.
Summing up Pandas DataFrame to List
And that’s 5 ways to convert Pandas DataFrame to a list. You’ve learned how to convert a single row or column to a 1-dimensional list, and also how to convert the entire DataFrame to a list of lists, a list of dictionaries, or a list of tuples.
We have to admit that the syntax isn’t consistent. For example, some functions are named with an underscore, such as to_records()
and to_dict()
, while others aren’t, such as tolist()
. It can be a bit confusing to beginners, and we hope to see these inconsistencies addressed in future Pandas releases.
What’s your favorite way to convert a Pandas DataFrame to a Python list? Let us know in the comment section below.